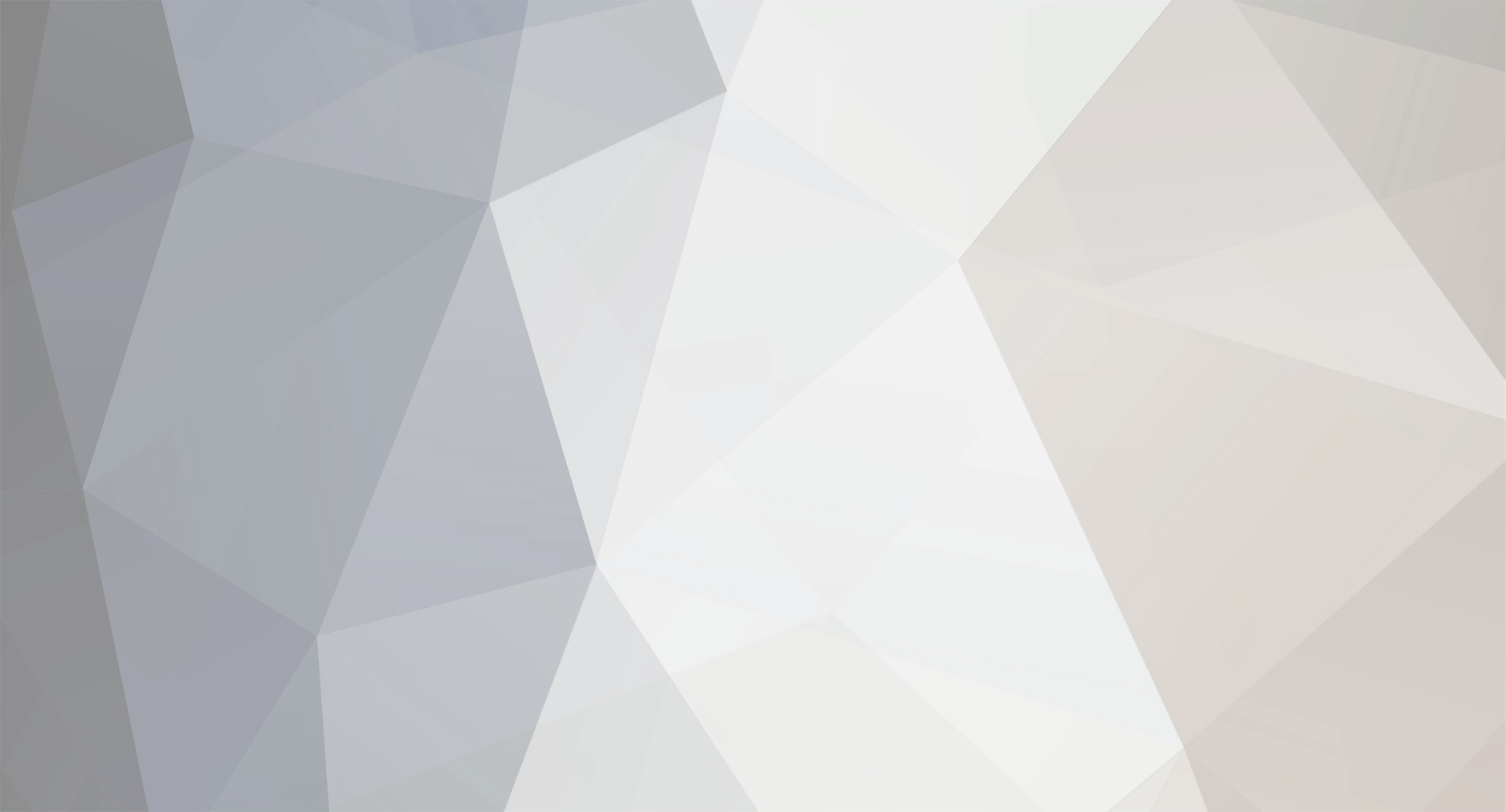
GeorgeTsak
Members-
Posts
17 -
Joined
-
Last visited
Everything posted by GeorgeTsak
-
I used an OBJ model in my mod to render a block. While it renders ok, I can't get it to render when being hold or when in inventory. I have tried registering it in many ways but it still doesn't work. The item in hand appears as purple/black cube and in inventory as a 2d purple/black texture. (There is no error in console): ModelLoader.setCustomModelResourceLocation(ItemSnail, 0, new ModelResourceLocation(ItemSnail.getRegistryName().toString()+".obj", "inventory")); ModelLoader.setCustomModelResourceLocation(ItemSnail, 0, new ModelResourceLocation(ItemSnail.getRegistryName().toString()+".obj")); ModelLoader.setCustomModelResourceLocation(ItemSnail, 0, new ModelResourceLocation(ItemSnail.getRegistryName().toString()+".obj", "normal")); ModelLoader.setCustomModelResourceLocation(ItemSnail, 0, new ModelResourceLocation(ItemSnail.getRegistryName().toString(), "inventory")); ModelLoader.setCustomModelResourceLocation(ItemSnail, 0, new ModelResourceLocation(ItemSnail.getRegistryName().toString())); ModelLoader.setCustomModelResourceLocation(ItemSnail, 0, new ModelResourceLocation(ItemSnail.getRegistryName().toString(), "normal")); (I am not into blockstates that much) Here is my JSON file in blockstates: What am I doing wrong?
-
I recently used Templates to spawn structures using the Template. I was impressed at how easy it is to use whith the structure block format. (NBT file). But I have a problem where when the structure spawns some torches they drop and are not placed on the walls. It seems to affect specific torches. I used MCEDIT to export the structure as vanilla only allows 32x32 structures. My structure size is 109x41x51. I know the size is big but I don't think that's the problem. Only some torches are affected by this.(Even paintings, flowerpots, chests with items) spawn ok. (Maybe MCEDIT has an issue when creating the NBT file?). Here's my code: (the #randomRot method just returns a random Rotation) Template template = getTemplate("my_structure", world); PlacementSettings placementsettings = (new PlacementSettings()).setMirror(Mirror.NONE).setRotation(randomRot(rand)).setIgnoreEntities(false).setChunk((ChunkPos)null).setReplacedBlock((Block)null).setIgnoreStructureBlock(false); if (template != null){ template.addBlocksToWorld(world, new BlockPos(randX, finalY, randZ), placementsettings); System.out.println("Created structure at " + randX + " // " + randZ); } And the #getTemplate method: private Template getTemplate(String fileName, World world){ WorldServer worldserver = (WorldServer)world; MinecraftServer minecraftserver = world.getMinecraftServer(); TemplateManager templatemanager = worldserver.getStructureTemplateManager(); return templatemanager.get(minecraftserver, new ResourceLocation(MyMod.MODID, fileName)); }
-
Thank you very much for your quick and helpful responses!
-
Maybe I didn't explain it well. So let's say I have a field in conf file that determines if a custom block will be registered or not. Let's suppose that Client has this field set to false(Block won't be registered) but Server has it set to true. When the Client tries to connect it will receive the config values and see that this specific value is different. So in order to get this block registered it will have to restart. But uppon loading won't the client load the old configuration file again? So the block will still be unregistered and the same thing will repeat. So as it seems the config file will have to be edited....
-
I think I got it. (SERVER PlayerLogsIn) -> Send Package to Client (Client Received Package) -> Store temporary in static fields But let's suppose that a certain config option e.g. registration of a block is changed. Then the player would have to restart to apply changes but those changes would be lost... (I do not have such a problem, I just want to learn if such a problem can be resolved)
-
Recently I added a config file to my mod and it works perfectly when in single player mode. However when I start a server and a player tries to join in with different config than the config of server, things don't work as they should. I understand I must use packets to send a packet to client and update it's config values. But... how do I do this. I already have used packets for other things like potion effects, removal of blocks and so on, but I do not know how to do this.
-
Ok finally I got it. Aparrently the read method of Template does not work with the new Structure NBT of the 1.10+ So what I did was create a custom class copying the read code from 1.10. Now it works perfectly even if code is messed up a bit.
-
OK since func_190008_d does not exist,I used the Template#read method like this: public class NBTBuilding { NBTTagCompound nbtTagCompound; public NBTBuilding(String filename){ try { InputStream is = Schematic.class.getResourceAsStream("/assets/modname/schematics/" + filename + ".nbt"); this.nbtTagCompound = CompressedStreamTools.readCompressed(is); is.close(); } catch (Exception e){ FMLLog.warning("Error occurred while reading " + filename + ".nbt"); FMLLog.warning(String.valueOf(e)); } } public void createBuilding(World world, int x, int y, int z){ //System.out.println("Requested to create a building at " + x + ", " + y + ", " + z); Template template = ((WorldServer)(world)).getStructureTemplateManager().getTemplate(world.getMinecraftServer(), new ResourceLocation(ModName.MODID, "name")); template.read(nbtTagCompound); template.addBlocksToWorldChunk(world, new BlockPos(x, y, z), new PlacementSettings()); } } Well the structure generates in the correct shape however the blocks are messed up... Not a single block is correct. Eg instead of Stone it uses Lava. Checking here http://minecraft.gamepedia.com/Structure_block_file_format I see that my NBT file has the correct format. Any clue?
-
I used MCEdit and exported a building as .NBT and I was able to get BlockPos's as well the block using Block#getBlockFromName() but I have no idea how to get the BlockState properties. I know where they are stored but I don't know how to convert them to Block State. See the picture. For example at the first index in the Properties Compount Tag it has a property snowy: false. What I would use is Blocks.GRASS.getDefaultState().withProperty(BlockGrass.SNOWY, false) Searching the minecraft's source code I found some classes like IBlockStatePalette that I suspect they can transform this but I do not know how to use it
-
Hi! I wanted to introduce some structure generation in my mod but I have problem with the new BlockStates... So in previous versions I would simply use a .schematic to Java converter but now there is no such tool for 1.9.4. Anyway what I would like to learn is, how can I make a structure that I made in minecraft be spawned? For example I make a house in vanilla Minecraft -> Export Structure with MCEdit -> ? Then? Is there any API that can read .schematic files directly or any piece of code that can do that?
-
Hi. I was wondering how (and if) I can resize a specific box in an entity's model. To put it in a perspective imagine a creeper that is about to explode. It expands. For example I want to make only the body to expand... (I do not want to apply this to a creeper) Let's take an example code... public class ModelExample extends ModelBase { ModelRenderer box1; ModelRenderer box2; public ModelExample() { box1 = new ModelRenderer(this, 0, 0); box1.addBox(0F, 0F, 0F, 1, 1, 1); box2.setRotationPoint(0F, 0F, 0F); box1.setTextureSize(32, 32); box1.mirror = true; setRotation(box1, 0F, 0F, 0F); box2 = new ModelRenderer(this, 18, 0); box2.addBox(1F, 0F, 0F, 1, 1, 1); box2.setRotationPoint(0F, 0F, 0F); box2.setTextureSize(32, 32); box2.mirror = true; setRotation(box2, 0F, 0F, 0F); } public void render(Entity entity, float f, float f1, float f2, float f3, float f4, float f5) { super.render(entity, f, f1, f2, f3, f4, f5); setRotationAngles(f, f1, f2, f3, f4, f5, entity); box1.render(f5); box2.render(f5); } private void setRotation(ModelRenderer model, float x, float y, float z) { model.rotateAngleX = x; model.rotateAngleY = y; model.rotateAngleZ = z; } public void setRotationAngles(float f, float f1, float f2, float f3, float f4, float f5, Entity entity) { super.setRotationAngles(f, f1, f2, f3, f4, f5, entity); } How can I edit the size/dimensions of box2?
-
Ok so I created this OPCooldownBar cb = new OPCooldownBar(); @SubscribeEvent public void RenderGameOverlayEvent(RenderGameOverlayEvent event){ cb.render(cooldown, cooldownMax); } and here is the OPCooldownBar class public class OPCooldownBar extends Gui { public OPCooldownBar() { super(); } int offsetX = 5; int y = 223; int xSize = 80; int green = Color.GREEN.getRGB(); int red = Color.RED.getRGB(); public void render(int cooldown, int cooldownMax){ int i = (int)getPercentage(cooldown, cooldownMax); this.drawRect(offsetX, y, offsetX + xSize - i, y + 3, getColor(i)); } private int getColor(int i) { if(i == 0){ return green; } return red; } public float getPercentage(int n, int total) { float proportion = ((float) n) / ((float) total); return proportion * xSize; } } Everything works fine except that the GUI colors act a little strange and that 2 bars instead of one are showing (See "circled" areas):
-
Hi. I would like to ask how I can add a GUI in the normal playing screen. I want to add a bar like the Hunger/Health bar which is always shown and it does not pause the game. I tried with GuiScreen but it pauses the game and it disables controls like movement.
-
Hi, I am trying to add a Poison Potion in my recipe for my item but it does work for all potions. I searched already the minecraftforge forums for answer and found this. Let's pretend I want to get an apple with a Poison Potion and a stick. ItemStack potion = new ItemStack(Items.POTIONITEM); PotionUtils.addPotionToItemStack(potion, PotionTypes.POISON); GameRegistry.addShapedRecipe(new ItemStack(Items.APPLE), new Object[]{"P ", "S ", 'S', Items.STICK, 'P', potion}); any idea?
-
Thanks everyone for their help. I just copied the method from the EntityRenderer and changed some variables! Now It works perfectly! ;D
-
Hey, I am making a mod and I want to add a Gun that will cause damage to entities or other players. However I cannot find how I can get the entity the player is looking at. I tried public ActionResult<ItemStack> onItemRightClick(ItemStack itemStackIn, World worldIn, EntityPlayer playerIn, EnumHand hand) { RayTraceResult mop = Minecraft.getMinecraft().objectMouseOver; if(mop != null){ System.out.println(mop.entityHit); } } but it doesn't work if the player is away from the entity (It displayes the entity when the player has the "Village-Player trade" distance). Does anyone know how I can make it?