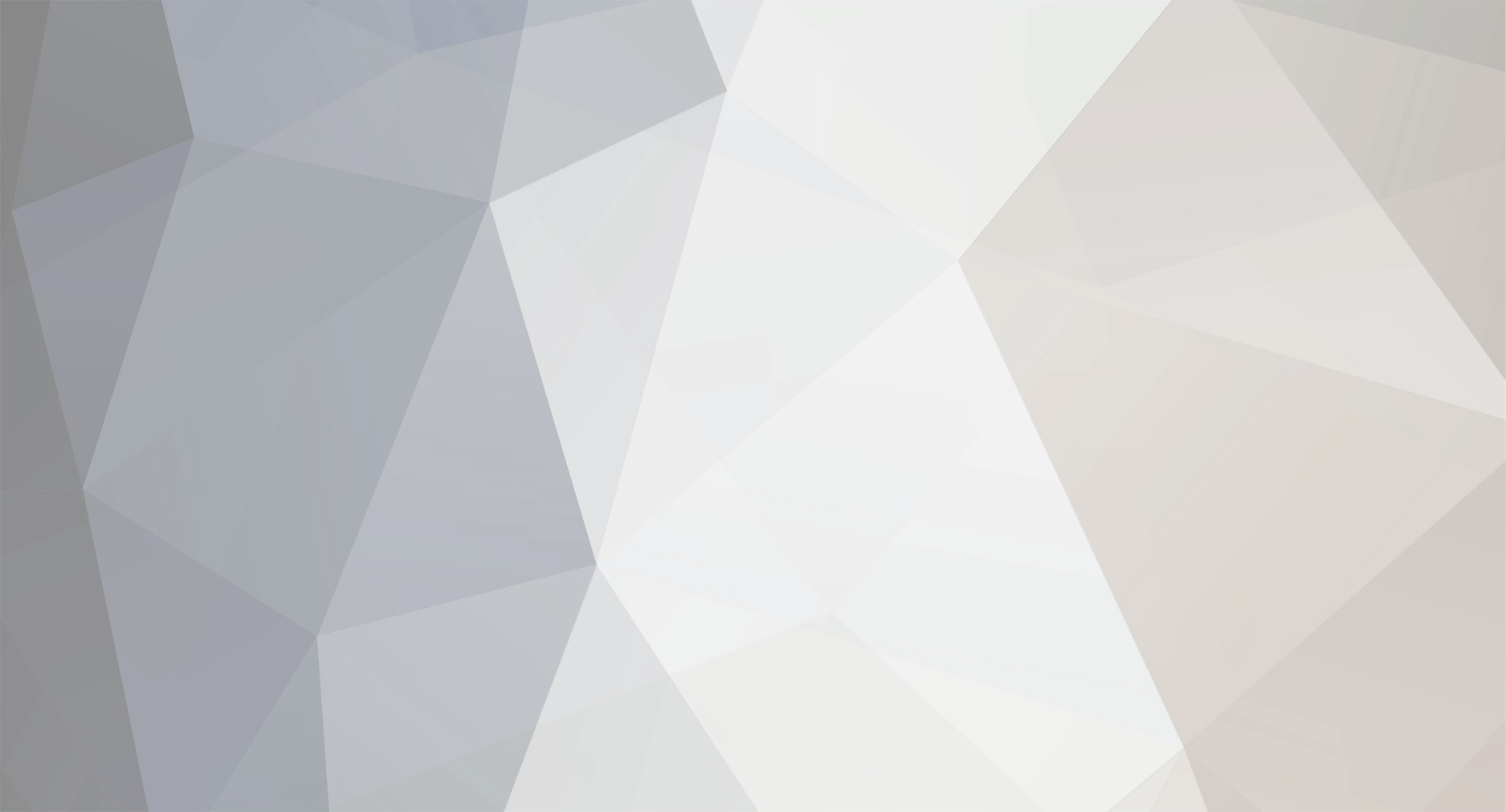
ThatCreepyUncle
Members-
Posts
27 -
Joined
-
Last visited
Converted
-
Gender
Undisclosed
-
Personal Text
I am new!
ThatCreepyUncle's Achievements

Tree Puncher (2/8)
0
Reputation
-
THANK YOU SO MUCH!!!! IT FINALLY WORKED!
-
1) I moved it to the LoadMeta Items to the preInit() method 2) I added super.preInit() to the init method in my client proxy! 3) I dont get the because, am I wrong but don't I need those to registry the models of my Items? So if I didn't have them would it not register the Item model? Do I remove them or change them, I am confused.
-
Sorry, didn't see the second page, so I thought they were not posting
-
Oh sorry: ModItems: package com.thatcreepyuncle.logicalTech.core; import java.util.ArrayList; import com.thatcreepyuncle.logicalTech.item.Circuit; import com.thatcreepyuncle.logicalTech.item.RemoteEnderporter; import com.thatcreepyuncle.logicalTech.item.RemoteSwitch; import net.minecraft.client.Minecraft; import net.minecraft.client.renderer.block.model.ModelResourceLocation; import net.minecraft.item.Item; import net.minecraftforge.fml.common.registry.GameRegistry; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; public class ModItems { public static ArrayList<Item> items; public static Item circuit; public static Item remote_switch; public static Item remote_enderporter; public static void preInit() { items = new ArrayList<Item>(); circuit = new Circuit(); GameRegistry.register(circuit); remote_switch = new RemoteSwitch(); GameRegistry.register(remote_switch); remote_enderporter = new RemoteEnderporter(); items.add(remote_enderporter); for (Item item : items) { GameRegistry.register(item); } } @SideOnly(Side.CLIENT) public static void init() { registerItemRenderer(circuit, 0, "toolCircuit"); registerItemRenderer(circuit, 1, "redstoneCircuit"); registerItemRenderer(circuit, 2, "motherboardCircuit"); registerItemRenderer(remote_switch, 0, "remote_switch"); registerItemRenderer(remote_switch, 1, "remote_switch_activated"); for (Item item : items) { registerItemRenderer(item); } } public static void registerItemRenderer(Item item) { Minecraft.getMinecraft().getRenderItem().getItemModelMesher() .register(item, 0, new ModelResourceLocation(item.getRegistryName(), "inventory")); } public static void registerItemRenderer(Item item, int meta, String fileName) { Minecraft.getMinecraft().getRenderItem().getItemModelMesher() .register(item, meta, new ModelResourceLocation((fileName), "inventory")); } }
-
Sorry I didn't post sooner but here is the new code. Client Proxy package com.thatcreepyuncle.logicalTech.core; import net.minecraft.client.renderer.block.model.ModelBakery; import net.minecraft.client.renderer.block.model.ModelResourceLocation; import net.minecraft.util.ResourceLocation; import net.minecraftforge.client.model.ModelLoader; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; public class ClientProxy extends CommonProxy{ @Override public void init() { ModItems.init(); loadMetaItems(); } @Override public void preInit() { ModBlocks.preInit(); ModItems.preInit(); } @Override public void loadMetaItems() { System.out.println("Loading Meta Items"); ModelLoader.setCustomModelResourceLocation(ModItems.circuit, 0, new ModelResourceLocation("ltech:toolCircuit")); ModelLoader.setCustomModelResourceLocation(ModItems.circuit, 1, new ModelResourceLocation("ltech:redstoneCircuit")); ModelLoader.setCustomModelResourceLocation(ModItems.circuit, 2, new ModelResourceLocation("ltech:motherboardCircuit")); ModelLoader.setCustomModelResourceLocation(ModItems.remote_switch, 0, new ModelResourceLocation("ltech:remote_switch")); ModelLoader.setCustomModelResourceLocation(ModItems.remote_switch, 1, new ModelResourceLocation("ltech:remote_switch_activated")); } } Common Proxy: package com.thatcreepyuncle.logicalTech.core; import com.thatcreepyuncle.logicalTech.LogicalTech; import com.thatcreepyuncle.logicalTech.tileEntity.TileEntityCircuitWorkbench; import com.thatcreepyuncle.logicalTech.tileEntity.TileEntityComputer; import net.minecraft.client.renderer.block.model.ModelBakery; import net.minecraft.util.ResourceLocation; import net.minecraftforge.fml.common.network.NetworkRegistry; import net.minecraftforge.fml.common.registry.GameRegistry; public class CommonProxy { public void preInit() { ModBlocks.preInit(); ModItems.preInit(); } public void init() { GameRegistry.registerTileEntity(TileEntityComputer.class, "computer"); GameRegistry.registerTileEntity(TileEntityCircuitWorkbench.class, "Circuit_Workbench"); NetworkRegistry.INSTANCE.registerGuiHandler(LogicalTech.instance, new GuiHandler()); } public static void postInit() { } public void loadMetaItems() { } } Mod Class package com.thatcreepyuncle.logicalTech; import com.thatcreepyuncle.logicalTech.core.CommonProxy; import com.thatcreepyuncle.logicalTech.network.CraftingMessage; import com.thatcreepyuncle.logicalTech.util.ModCreativeTabs; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.common.Mod.EventHandler; import net.minecraftforge.fml.common.Mod.Instance; import net.minecraftforge.fml.common.SidedProxy; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; import net.minecraftforge.fml.common.network.NetworkRegistry; import net.minecraftforge.fml.common.network.simpleimpl.SimpleNetworkWrapper; import net.minecraftforge.fml.relauncher.Side; @Mod(modid = LogicalTech.MODID, version = LogicalTech.VERSION) public class LogicalTech { public static final String MODID = "ltech"; public static final String VERSION = "1.0"; @Instance(MODID) public static LogicalTech instance; @SidedProxy(clientSide="com.thatcreepyuncle.logicalTech.core.ClientProxy", serverSide="com.thatcreepyuncle.logicalTech.core.ServerProxy") public static CommonProxy proxy; @EventHandler public void preInit(FMLPreInitializationEvent e) { ModCreativeTabs tabs = new ModCreativeTabs(); proxy.preInit(); } @EventHandler public void init(FMLInitializationEvent e) { proxy.init(); } @EventHandler public void postInit(FMLPostInitializationEvent e) { proxy.postInit(); } } Item Class: package com.thatcreepyuncle.logicalTech.item; import java.util.List; import com.thatcreepyuncle.logicalTech.util.ModCreativeTabs; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; public class Circuit extends Item{ public Circuit() { super(); this.setHasSubtypes(true); this.setRegistryName("circuit"); this.setCreativeTab(ModCreativeTabs.GENERAL); this.setUnlocalizedName("circuit"); } @Override public String getUnlocalizedName(ItemStack stack) { String name = EnumCircuit.values()[stack.getItemDamage()].getUnlocalizedName(); return "circuit_" + name; } @Override public void getSubItems(Item itemIn, CreativeTabs tab, List subItems) { for (int i = 0; i < 3; i++) { subItems.add(new ItemStack(itemIn, 1, i)); } } } Log: 2016-07-12 16:52:16,122 WARN Unable to instantiate org.fusesource.jansi.WindowsAnsiOutputStream 2016-07-12 16:52:16,127 WARN Unable to instantiate org.fusesource.jansi.WindowsAnsiOutputStream [16:52:16] [main/INFO] [GradleStart]: Extra: [] [16:52:16] [main/INFO] [GradleStart]: Running with arguments: [--userProperties, {}, --assetsDir, C:/Users/Bob/.gradle/caches/minecraft/assets, --assetIndex, 1.9, --accessToken{REDACTED}, --version, 1.9.4, --tweakClass, net.minecraftforge.fml.common.launcher.FMLTweaker, --tweakClass, net.minecraftforge.gradle.tweakers.CoremodTweaker] [16:52:16] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.FMLTweaker [16:52:16] [main/INFO] [LaunchWrapper]: Using primary tweak class name net.minecraftforge.fml.common.launcher.FMLTweaker [16:52:16] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.gradle.tweakers.CoremodTweaker [16:52:16] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLTweaker [16:52:16] [main/INFO] [FML]: Forge Mod Loader version 12.17.0.1976 for Minecraft 1.9.4 loading [16:52:16] [main/INFO] [FML]: Java is Java HotSpot(TM) 64-Bit Server VM, version 1.8.0_65, running on Windows 10:amd64:10.0, installed at C:\Program Files\Java\jdk1.8.0_66\jre [16:52:16] [main/INFO] [FML]: Managed to load a deobfuscated Minecraft name- we are in a deobfuscated environment. Skipping runtime deobfuscation [16:52:16] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.gradle.tweakers.CoremodTweaker [16:52:16] [main/INFO] [GradleStart]: Injecting location in coremod net.minecraftforge.fml.relauncher.FMLCorePlugin [16:52:16] [main/INFO] [GradleStart]: Injecting location in coremod net.minecraftforge.classloading.FMLForgePlugin [16:52:16] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.FMLInjectionAndSortingTweaker [16:52:16] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.FMLDeobfTweaker [16:52:16] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.gradle.tweakers.AccessTransformerTweaker [16:52:16] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLInjectionAndSortingTweaker [16:52:16] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLInjectionAndSortingTweaker [16:52:16] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.relauncher.CoreModManager$FMLPluginWrapper [16:52:16] [main/ERROR] [FML]: The binary patch set is missing. Either you are in a development environment, or things are not going to work! [16:52:19] [main/ERROR] [FML]: FML appears to be missing any signature data. This is not a good thing [16:52:19] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.relauncher.CoreModManager$FMLPluginWrapper [16:52:19] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLDeobfTweaker [16:52:22] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.gradle.tweakers.AccessTransformerTweaker [16:52:22] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.TerminalTweaker [16:52:22] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.TerminalTweaker [16:52:22] [main/INFO] [LaunchWrapper]: Launching wrapped minecraft {net.minecraft.client.main.Main} 2016-07-12 16:52:23,944 WARN Unable to instantiate org.fusesource.jansi.WindowsAnsiOutputStream 2016-07-12 16:52:24,008 WARN Unable to instantiate org.fusesource.jansi.WindowsAnsiOutputStream 2016-07-12 16:52:24,013 WARN Unable to instantiate org.fusesource.jansi.WindowsAnsiOutputStream [16:52:24] [Client thread/INFO]: Setting user: Player391 [16:52:32] [Client thread/INFO]: LWJGL Version: 2.9.4 [16:52:34] [Client thread/INFO] [sTDOUT]: [net.minecraftforge.fml.client.SplashProgress:start:202]: ---- Minecraft Crash Report ---- // I'm sorry, Dave. Time: 7/12/16 4:52 PM Description: Loading screen debug info This is just a prompt for computer specs to be printed. THIS IS NOT A ERROR A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- System Details -- Details: Minecraft Version: 1.9.4 Operating System: Windows 10 (amd64) version 10.0 Java Version: 1.8.0_65, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 865528912 bytes (825 MB) / 1038876672 bytes (990 MB) up to 1038876672 bytes (990 MB) JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: Loaded coremods (and transformers): GL info: ' Vendor: 'ATI Technologies Inc.' Version: '4.5.13416 Compatibility Profile Context 15.300.1025.1001' Renderer: 'AMD Radeon R7 200 Series' [16:52:34] [Client thread/INFO] [FML]: MinecraftForge v12.17.0.1976 Initialized [16:52:34] [Client thread/INFO] [FML]: Replaced 232 ore recipes [16:52:35] [Client thread/INFO] [FML]: Found 0 mods from the command line. Injecting into mod discoverer [16:52:35] [Client thread/INFO] [FML]: Searching C:\Users\Bob\Desktop\LogicalTech 1.9.4\run\mods for mods [16:52:35] [Client thread/INFO] [ltech]: Mod ltech is missing the required element 'name'. Substituting ltech [16:52:37] [Client thread/INFO] [FML]: Forge Mod Loader has identified 4 mods to load [16:52:38] [Client thread/INFO] [FML]: Attempting connection with missing mods [mcp, FML, Forge, ltech] at CLIENT [16:52:38] [Client thread/INFO] [FML]: Attempting connection with missing mods [mcp, FML, Forge, ltech] at SERVER [16:52:39] [Client thread/INFO]: Reloading ResourceManager: Default, FMLFileResourcePack:Forge Mod Loader, FMLFileResourcePack:Minecraft Forge, FMLFileResourcePack:ltech [16:52:39] [Client thread/INFO] [FML]: Processing ObjectHolder annotations [16:52:39] [Client thread/INFO] [FML]: Found 418 ObjectHolder annotations [16:52:39] [Client thread/INFO] [FML]: Identifying ItemStackHolder annotations [16:52:39] [Client thread/INFO] [FML]: Found 0 ItemStackHolder annotations [16:52:39] [Client thread/INFO] [FML]: Configured a dormant chunk cache size of 0 [16:52:39] [Forge Version Check/INFO] [ForgeVersionCheck]: [Forge] Starting version check at http://files.minecraftforge.net/maven/net/minecraftforge/forge/promotions_slim.json [16:52:39] [Client thread/INFO] [sTDOUT]: [com.thatcreepyuncle.logicalTech.core.ClientProxy:loadMetaItems:26]: Loading Meta Items [16:52:39] [Client thread/INFO] [FML]: Applying holder lookups [16:52:40] [Client thread/INFO] [FML]: Holder lookups applied [16:52:40] [Client thread/INFO] [FML]: Injecting itemstacks [16:52:40] [Client thread/INFO] [FML]: Itemstack injection complete [16:52:40] [Forge Version Check/INFO] [ForgeVersionCheck]: [Forge] Found status: UP_TO_DATE Target: null [16:52:46] [sound Library Loader/INFO]: Starting up SoundSystem... [16:52:46] [Thread-8/INFO]: Initializing LWJGL OpenAL [16:52:46] [Thread-8/INFO]: (The LWJGL binding of OpenAL. For more information, see http://www.lwjgl.org) [16:52:47] [Thread-8/INFO]: OpenAL initialized. [16:52:47] [sound Library Loader/INFO]: Sound engine started [16:52:56] [Client thread/INFO] [FML]: Max texture size: 16384 [16:52:56] [Client thread/INFO]: Created: 16x16 textures-atlas [16:52:59] [Client thread/INFO] [FML]: Injecting itemstacks [16:52:59] [Client thread/INFO] [FML]: Itemstack injection complete [16:52:59] [Client thread/INFO] [FML]: Forge Mod Loader has successfully loaded 4 mods [16:52:59] [Client thread/INFO]: Reloading ResourceManager: Default, FMLFileResourcePack:Forge Mod Loader, FMLFileResourcePack:Minecraft Forge, FMLFileResourcePack:ltech [16:53:04] [Client thread/INFO]: SoundSystem shutting down... [16:53:05] [Client thread/WARN]: Author: Paul Lamb, www.paulscode.com [16:53:05] [sound Library Loader/INFO]: Starting up SoundSystem... [16:53:05] [Thread-10/INFO]: Initializing LWJGL OpenAL [16:53:05] [Thread-10/INFO]: (The LWJGL binding of OpenAL. For more information, see http://www.lwjgl.org) [16:53:05] [Thread-10/INFO]: OpenAL initialized. [16:53:05] [sound Library Loader/INFO]: Sound engine started [16:53:12] [Client thread/INFO] [FML]: Max texture size: 16384 [16:53:13] [Client thread/INFO]: Created: 1024x512 textures-atlas [16:53:16] [Realms Notification Availability checker #1/INFO]: Could not authorize you against Realms server: Invalid session id [16:53:21] [server thread/INFO]: Starting integrated minecraft server version 1.9.4 [16:53:21] [server thread/INFO]: Generating keypair [16:53:21] [server thread/INFO] [FML]: Injecting existing block and item data into this server instance [16:53:21] [server thread/INFO] [FML]: Applying holder lookups [16:53:21] [server thread/INFO] [FML]: Holder lookups applied [16:53:22] [server thread/INFO] [FML]: Loading dimension 0 (World) (net.minecraft.server.integrated.IntegratedServer@3a4379a2) [16:53:22] [server thread/INFO] [FML]: Loading dimension 1 (World) (net.minecraft.server.integrated.IntegratedServer@3a4379a2) [16:53:22] [server thread/INFO] [FML]: Loading dimension -1 (World) (net.minecraft.server.integrated.IntegratedServer@3a4379a2) [16:53:22] [server thread/INFO]: Preparing start region for level 0 [16:53:23] [server thread/INFO]: Preparing spawn area: 48% [16:53:24] [server thread/INFO]: Changing view distance to 2, from 10 [16:53:27] [Netty Local Client IO #0/INFO] [FML]: Server protocol version 2 [16:53:27] [Netty Server IO #1/INFO] [FML]: Client protocol version 2 [16:53:27] [Netty Server IO #1/INFO] [FML]: Client attempting to join with 4 mods : [email protected],[email protected],[email protected],[email protected] [16:53:27] [Netty Local Client IO #0/INFO] [FML]: [Netty Local Client IO #0] Client side modded connection established [16:53:27] [server thread/INFO] [FML]: [server thread] Server side modded connection established [16:53:27] [server thread/INFO]: Player391[local:E:3fc8612c] logged in with entity id 447 at (269.4931499573029, 68.0, 219.54516940443818) [16:53:27] [server thread/INFO]: Player391 joined the game [16:53:28] [server thread/INFO]: Saving and pausing game... [16:53:28] [server thread/INFO]: Saving chunks for level 'World'/Overworld [16:53:28] [pool-2-thread-1/WARN]: Couldn't look up profile properties for com.mojang.authlib.GameProfile@68af052c[id=e879cd92-2843-3668-b364-5d9cff074bc0,name=Player391,properties={},legacy=false] com.mojang.authlib.exceptions.AuthenticationException: The client has sent too many requests within a certain amount of time at com.mojang.authlib.yggdrasil.YggdrasilAuthenticationService.makeRequest(YggdrasilAuthenticationService.java:65) ~[YggdrasilAuthenticationService.class:?] at com.mojang.authlib.yggdrasil.YggdrasilMinecraftSessionService.fillGameProfile(YggdrasilMinecraftSessionService.java:175) [YggdrasilMinecraftSessionService.class:?] at com.mojang.authlib.yggdrasil.YggdrasilMinecraftSessionService$1.load(YggdrasilMinecraftSessionService.java:59) [YggdrasilMinecraftSessionService$1.class:?] at com.mojang.authlib.yggdrasil.YggdrasilMinecraftSessionService$1.load(YggdrasilMinecraftSessionService.java:56) [YggdrasilMinecraftSessionService$1.class:?] at com.google.common.cache.LocalCache$LoadingValueReference.loadFuture(LocalCache.java:3524) [guava-17.0.jar:?] at com.google.common.cache.LocalCache$Segment.loadSync(LocalCache.java:2317) [guava-17.0.jar:?] at com.google.common.cache.LocalCache$Segment.lockedGetOrLoad(LocalCache.java:2280) [guava-17.0.jar:?] at com.google.common.cache.LocalCache$Segment.get(LocalCache.java:2195) [guava-17.0.jar:?] at com.google.common.cache.LocalCache.get(LocalCache.java:3934) [guava-17.0.jar:?] at com.google.common.cache.LocalCache.getOrLoad(LocalCache.java:3938) [guava-17.0.jar:?] at com.google.common.cache.LocalCache$LocalLoadingCache.get(LocalCache.java:4821) [guava-17.0.jar:?] at com.google.common.cache.LocalCache$LocalLoadingCache.getUnchecked(LocalCache.java:4827) [guava-17.0.jar:?] at com.mojang.authlib.yggdrasil.YggdrasilMinecraftSessionService.fillProfileProperties(YggdrasilMinecraftSessionService.java:165) [YggdrasilMinecraftSessionService.class:?] at net.minecraft.client.Minecraft.getProfileProperties(Minecraft.java:3043) [Minecraft.class:?] at net.minecraft.client.resources.SkinManager$3.run(SkinManager.java:131) [skinManager$3.class:?] at java.util.concurrent.Executors$RunnableAdapter.call(Executors.java:511) [?:1.8.0_65] at java.util.concurrent.FutureTask.run(FutureTask.java:266) [?:1.8.0_65] at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1142) [?:1.8.0_65] at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:617) [?:1.8.0_65] at java.lang.Thread.run(Thread.java:745) [?:1.8.0_65] [16:53:28] [server thread/INFO]: Saving chunks for level 'World'/Nether [16:53:28] [server thread/INFO]: Saving chunks for level 'World'/The End [16:53:29] [server thread/WARN]: Can't keep up! Did the system time change, or is the server overloaded? Running 2175ms behind, skipping 43 tick(s) [16:53:32] [server thread/INFO]: Player391 has just earned the achievement [Taking Inventory] [16:53:32] [Client thread/INFO]: [CHAT] Player391 has just earned the achievement [Taking Inventory]
-
I removed It ,but it still Doesn't Work. The textures are black and purple still. Log(If it helps): 2016-07-10 12:28:37,998 WARN Unable to instantiate org.fusesource.jansi.WindowsAnsiOutputStream 2016-07-10 12:28:38,000 WARN Unable to instantiate org.fusesource.jansi.WindowsAnsiOutputStream [12:28:38] [main/INFO] [GradleStart]: Extra: [] [12:28:38] [main/INFO] [GradleStart]: Running with arguments: [--userProperties, {}, --assetsDir, C:/Users/Bob/.gradle/caches/minecraft/assets, --assetIndex, 1.9, --accessToken{REDACTED}, --version, 1.9.4, --tweakClass, net.minecraftforge.fml.common.launcher.FMLTweaker, --tweakClass, net.minecraftforge.gradle.tweakers.CoremodTweaker] [12:28:38] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.FMLTweaker [12:28:38] [main/INFO] [LaunchWrapper]: Using primary tweak class name net.minecraftforge.fml.common.launcher.FMLTweaker [12:28:38] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.gradle.tweakers.CoremodTweaker [12:28:38] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLTweaker [12:28:38] [main/INFO] [FML]: Forge Mod Loader version 12.17.0.1976 for Minecraft 1.9.4 loading [12:28:38] [main/INFO] [FML]: Java is Java HotSpot(TM) 64-Bit Server VM, version 1.8.0_65, running on Windows 10:amd64:10.0, installed at C:\Program Files\Java\jdk1.8.0_66\jre [12:28:38] [main/INFO] [FML]: Managed to load a deobfuscated Minecraft name- we are in a deobfuscated environment. Skipping runtime deobfuscation [12:28:38] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.gradle.tweakers.CoremodTweaker [12:28:38] [main/INFO] [GradleStart]: Injecting location in coremod net.minecraftforge.fml.relauncher.FMLCorePlugin [12:28:38] [main/INFO] [GradleStart]: Injecting location in coremod net.minecraftforge.classloading.FMLForgePlugin [12:28:38] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.FMLInjectionAndSortingTweaker [12:28:38] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.FMLDeobfTweaker [12:28:38] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.gradle.tweakers.AccessTransformerTweaker [12:28:38] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLInjectionAndSortingTweaker [12:28:38] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLInjectionAndSortingTweaker [12:28:38] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.relauncher.CoreModManager$FMLPluginWrapper [12:28:38] [main/ERROR] [FML]: The binary patch set is missing. Either you are in a development environment, or things are not going to work! [12:28:41] [main/ERROR] [FML]: FML appears to be missing any signature data. This is not a good thing [12:28:41] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.relauncher.CoreModManager$FMLPluginWrapper [12:28:41] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLDeobfTweaker [12:28:43] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.gradle.tweakers.AccessTransformerTweaker [12:28:43] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.TerminalTweaker [12:28:43] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.TerminalTweaker [12:28:43] [main/INFO] [LaunchWrapper]: Launching wrapped minecraft {net.minecraft.client.main.Main} 2016-07-10 12:28:44,026 WARN Unable to instantiate org.fusesource.jansi.WindowsAnsiOutputStream 2016-07-10 12:28:44,094 WARN Unable to instantiate org.fusesource.jansi.WindowsAnsiOutputStream 2016-07-10 12:28:44,102 WARN Unable to instantiate org.fusesource.jansi.WindowsAnsiOutputStream [12:28:44] [Client thread/INFO]: Setting user: Player512 [12:28:50] [Client thread/INFO]: LWJGL Version: 2.9.4 [12:28:53] [Client thread/INFO] [sTDOUT]: [net.minecraftforge.fml.client.SplashProgress:start:202]: ---- Minecraft Crash Report ---- // Hi. I'm Minecraft, and I'm a crashaholic. Time: 7/10/16 12:28 PM Description: Loading screen debug info This is just a prompt for computer specs to be printed. THIS IS NOT A ERROR A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- System Details -- Details: Minecraft Version: 1.9.4 Operating System: Windows 10 (amd64) version 10.0 Java Version: 1.8.0_65, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 772421544 bytes (736 MB) / 1038876672 bytes (990 MB) up to 1038876672 bytes (990 MB) JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: Loaded coremods (and transformers): GL info: ' Vendor: 'ATI Technologies Inc.' Version: '4.5.13416 Compatibility Profile Context 15.300.1025.1001' Renderer: 'AMD Radeon R7 200 Series' [12:28:53] [Client thread/INFO] [FML]: MinecraftForge v12.17.0.1976 Initialized [12:28:53] [Client thread/INFO] [FML]: Replaced 232 ore recipes [12:28:54] [Client thread/INFO] [FML]: Found 0 mods from the command line. Injecting into mod discoverer [12:28:54] [Client thread/INFO] [FML]: Searching C:\Users\Bob\Desktop\LogicalTech 1.9.4\run\mods for mods [12:28:54] [Client thread/INFO] [ltech]: Mod ltech is missing the required element 'name'. Substituting ltech [12:28:55] [Client thread/INFO] [FML]: Forge Mod Loader has identified 4 mods to load [12:28:55] [Client thread/INFO] [FML]: Attempting connection with missing mods [mcp, FML, Forge, ltech] at CLIENT [12:28:55] [Client thread/INFO] [FML]: Attempting connection with missing mods [mcp, FML, Forge, ltech] at SERVER [12:28:57] [Client thread/INFO]: Reloading ResourceManager: Default, FMLFileResourcePack:Forge Mod Loader, FMLFileResourcePack:Minecraft Forge, FMLFileResourcePack:ltech [12:28:57] [Client thread/INFO] [FML]: Processing ObjectHolder annotations [12:28:57] [Client thread/INFO] [FML]: Found 418 ObjectHolder annotations [12:28:57] [Client thread/INFO] [FML]: Identifying ItemStackHolder annotations [12:28:57] [Client thread/INFO] [FML]: Found 0 ItemStackHolder annotations [12:28:57] [Client thread/INFO] [FML]: Configured a dormant chunk cache size of 0 [12:28:57] [Forge Version Check/INFO] [ForgeVersionCheck]: [Forge] Starting version check at http://files.minecraftforge.net/maven/net/minecraftforge/forge/promotions_slim.json [12:28:57] [Forge Version Check/INFO] [ForgeVersionCheck]: [Forge] Found status: UP_TO_DATE Target: null [12:28:57] [Client thread/INFO] [FML]: Applying holder lookups [12:28:57] [Client thread/INFO] [FML]: Holder lookups applied [12:28:57] [Client thread/INFO] [FML]: Injecting itemstacks [12:28:57] [Client thread/INFO] [FML]: Itemstack injection complete [12:29:03] [sound Library Loader/INFO]: Starting up SoundSystem... [12:29:03] [Thread-8/INFO]: Initializing LWJGL OpenAL [12:29:03] [Thread-8/INFO]: (The LWJGL binding of OpenAL. For more information, see http://www.lwjgl.org) [12:29:03] [Thread-8/INFO]: OpenAL initialized. [12:29:03] [sound Library Loader/INFO]: Sound engine started [12:29:10] [Client thread/INFO] [FML]: Max texture size: 16384 [12:29:10] [Client thread/INFO]: Created: 16x16 textures-atlas [12:29:13] [Client thread/INFO] [FML]: Injecting itemstacks [12:29:13] [Client thread/INFO] [FML]: Itemstack injection complete [12:29:13] [Client thread/INFO] [FML]: Forge Mod Loader has successfully loaded 4 mods [12:29:13] [Client thread/INFO]: Reloading ResourceManager: Default, FMLFileResourcePack:Forge Mod Loader, FMLFileResourcePack:Minecraft Forge, FMLFileResourcePack:ltech [12:29:18] [Client thread/INFO]: SoundSystem shutting down... [12:29:19] [Client thread/WARN]: Author: Paul Lamb, www.paulscode.com [12:29:19] [sound Library Loader/INFO]: Starting up SoundSystem... [12:29:19] [Thread-10/INFO]: Initializing LWJGL OpenAL [12:29:19] [Thread-10/INFO]: (The LWJGL binding of OpenAL. For more information, see http://www.lwjgl.org) [12:29:19] [Thread-10/INFO]: OpenAL initialized. [12:29:19] [sound Library Loader/INFO]: Sound engine started [12:29:25] [Client thread/INFO] [FML]: Max texture size: 16384 [12:29:25] [Client thread/INFO]: Created: 1024x512 textures-atlas [12:29:27] [Realms Notification Availability checker #1/INFO]: Could not authorize you against Realms server: Invalid session id [12:30:16] [server thread/INFO]: Starting integrated minecraft server version 1.9.4 [12:30:16] [server thread/INFO]: Generating keypair [12:30:16] [server thread/INFO] [FML]: Injecting existing block and item data into this server instance [12:30:16] [server thread/INFO] [FML]: Applying holder lookups [12:30:16] [server thread/INFO] [FML]: Holder lookups applied [12:30:16] [server thread/INFO] [FML]: Loading dimension 0 (World) (net.minecraft.server.integrated.IntegratedServer@7023b082) [12:30:17] [server thread/INFO] [FML]: Loading dimension 1 (World) (net.minecraft.server.integrated.IntegratedServer@7023b082) [12:30:17] [server thread/INFO] [FML]: Loading dimension -1 (World) (net.minecraft.server.integrated.IntegratedServer@7023b082) [12:30:17] [server thread/INFO]: Preparing start region for level 0 [12:30:18] [server thread/INFO]: Preparing spawn area: 58% [12:30:19] [server thread/INFO]: Changing view distance to 2, from 10 [12:30:21] [Netty Local Client IO #0/INFO] [FML]: Server protocol version 2 [12:30:21] [Netty Server IO #1/INFO] [FML]: Client protocol version 2 [12:30:21] [Netty Server IO #1/INFO] [FML]: Client attempting to join with 4 mods : [email protected],[email protected],[email protected],[email protected] [12:30:21] [Netty Local Client IO #0/INFO] [FML]: [Netty Local Client IO #0] Client side modded connection established [12:30:21] [server thread/INFO] [FML]: [server thread] Server side modded connection established [12:30:21] [server thread/INFO]: Player512[local:E:0a945a5e] logged in with entity id 454 at (267.02422565986575, 67.0, 219.49761608486688) [12:30:21] [server thread/INFO]: Player512 joined the game [12:30:22] [server thread/INFO]: Saving and pausing game... [12:30:22] [server thread/INFO]: Saving chunks for level 'World'/Overworld [12:30:23] [server thread/INFO]: Saving chunks for level 'World'/Nether [12:30:23] [server thread/INFO]: Saving chunks for level 'World'/The End [12:30:23] [pool-2-thread-1/WARN]: Couldn't look up profile properties for com.mojang.authlib.GameProfile@7f6f03fe[id=4296a45d-fd1d-3a8b-9f25-0f078d10cc6b,name=Player512,properties={},legacy=false] com.mojang.authlib.exceptions.AuthenticationException: The client has sent too many requests within a certain amount of time at com.mojang.authlib.yggdrasil.YggdrasilAuthenticationService.makeRequest(YggdrasilAuthenticationService.java:65) ~[YggdrasilAuthenticationService.class:?] at com.mojang.authlib.yggdrasil.YggdrasilMinecraftSessionService.fillGameProfile(YggdrasilMinecraftSessionService.java:175) [YggdrasilMinecraftSessionService.class:?] at com.mojang.authlib.yggdrasil.YggdrasilMinecraftSessionService$1.load(YggdrasilMinecraftSessionService.java:59) [YggdrasilMinecraftSessionService$1.class:?] at com.mojang.authlib.yggdrasil.YggdrasilMinecraftSessionService$1.load(YggdrasilMinecraftSessionService.java:56) [YggdrasilMinecraftSessionService$1.class:?] at com.google.common.cache.LocalCache$LoadingValueReference.loadFuture(LocalCache.java:3524) [guava-17.0.jar:?] at com.google.common.cache.LocalCache$Segment.loadSync(LocalCache.java:2317) [guava-17.0.jar:?] at com.google.common.cache.LocalCache$Segment.lockedGetOrLoad(LocalCache.java:2280) [guava-17.0.jar:?] at com.google.common.cache.LocalCache$Segment.get(LocalCache.java:2195) [guava-17.0.jar:?] at com.google.common.cache.LocalCache.get(LocalCache.java:3934) [guava-17.0.jar:?] at com.google.common.cache.LocalCache.getOrLoad(LocalCache.java:3938) [guava-17.0.jar:?] at com.google.common.cache.LocalCache$LocalLoadingCache.get(LocalCache.java:4821) [guava-17.0.jar:?] at com.google.common.cache.LocalCache$LocalLoadingCache.getUnchecked(LocalCache.java:4827) [guava-17.0.jar:?] at com.mojang.authlib.yggdrasil.YggdrasilMinecraftSessionService.fillProfileProperties(YggdrasilMinecraftSessionService.java:165) [YggdrasilMinecraftSessionService.class:?] at net.minecraft.client.Minecraft.getProfileProperties(Minecraft.java:3043) [Minecraft.class:?] at net.minecraft.client.resources.SkinManager$3.run(SkinManager.java:131) [skinManager$3.class:?] at java.util.concurrent.Executors$RunnableAdapter.call(Executors.java:511) [?:1.8.0_65] at java.util.concurrent.FutureTask.run(FutureTask.java:266) [?:1.8.0_65] at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1142) [?:1.8.0_65] at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:617) [?:1.8.0_65] at java.lang.Thread.run(Thread.java:745) [?:1.8.0_65] [12:30:26] [server thread/INFO]: Player512 has just earned the achievement [Taking Inventory] [12:30:26] [Client thread/INFO]: [CHAT] Player512 has just earned the achievement [Taking Inventory] Is there any other code you want?
-
package com.thatcreepyuncle.logicalTech.core; import net.minecraft.client.renderer.block.model.ModelBakery; import net.minecraft.client.renderer.block.model.ModelResourceLocation; import net.minecraft.util.ResourceLocation; import net.minecraftforge.client.model.ModelLoader; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; public class ClientProxy extends CommonProxy{ @Override public void init() { loadMetaItems(); ModItems.init(); } @Override public void preInit() { super.preInit(); loadMetaItems(); } @Override public void loadMetaItems() { ModelLoader.setCustomModelResourceLocation(ModItems.circuit, 0, new ModelResourceLocation("ltech:toolCircuit")); ModelLoader.setCustomModelResourceLocation(ModItems.circuit, 1, new ModelResourceLocation("ltech:redstoneCircuit")); ModelLoader.setCustomModelResourceLocation(ModItems.circuit, 2, new ModelResourceLocation("ltech:motherboardCircuit")); ModelLoader.setCustomModelResourceLocation(ModItems.remote_switch, 0, new ModelResourceLocation("ltech:remote_switch")); ModelLoader.setCustomModelResourceLocation(ModItems.remote_switch, 1, new ModelResourceLocation("ltech:remote_switch_activated")); } }
-
Doesn't Work, Still no errors
-
Updated Client Proxy: package com.thatcreepyuncle.logicalTech.core; import net.minecraft.client.renderer.block.model.ModelBakery; import net.minecraft.client.renderer.block.model.ModelResourceLocation; import net.minecraft.util.ResourceLocation; import net.minecraftforge.client.model.ModelLoader; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; public class ClientProxy extends CommonProxy{ @Override public void init() { loadMetaItems(); ModItems.init(); } @Override public void preInit() { super.preInit(); } @Override public void loadMetaItems() { ModelLoader.setCustomModelResourceLocation(ModItems.circuit, 0, new ModelResourceLocation("ltech:toolCircuit")); ModelLoader.setCustomModelResourceLocation(ModItems.circuit, 1, new ModelResourceLocation("ltech:redstoneCircuit")); ModelLoader.setCustomModelResourceLocation(ModItems.circuit, 2, new ModelResourceLocation("ltech:motherboardCircuit")); ModelLoader.setCustomModelResourceLocation(ModItems.remote_switch, 0, new ModelResourceLocation("ltech:remote_switch")); ModelLoader.setCustomModelResourceLocation(ModItems.remote_switch, 1, new ModelResourceLocation("ltech:remote_switch_activated")); } } Updated Mod Items: package com.thatcreepyuncle.logicalTech.core; import java.util.ArrayList; import com.thatcreepyuncle.logicalTech.item.Circuit; import com.thatcreepyuncle.logicalTech.item.RemoteSwitch; import net.minecraft.client.Minecraft; import net.minecraft.client.renderer.block.model.ModelResourceLocation; import net.minecraft.item.Item; import net.minecraftforge.fml.common.registry.GameRegistry; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; public class ModItems { public static ArrayList<Item> items; public static Item circuit; public static Item remote_switch; public static void preInit() { items = new ArrayList<Item>(); circuit = new Circuit(); GameRegistry.register(circuit); remote_switch = new RemoteSwitch(); GameRegistry.register(remote_switch); for (Item item : items) { GameRegistry.register(item); } } @SideOnly(Side.CLIENT) public static void init() { registerItemRenderer(circuit, 0, "toolCircuit"); registerItemRenderer(circuit, 1, "redstoneCircuit"); registerItemRenderer(circuit, 2, "motherboardCircuit"); registerItemRenderer(remote_switch, 0, "remote_switch"); registerItemRenderer(remote_switch, 1, "remote_switch_activated"); for (Item item : items) { registerItemRenderer(item); } } public static void registerItemRenderer(Item item) { Minecraft.getMinecraft().getRenderItem().getItemModelMesher() .register(item, 0, new ModelResourceLocation(item.getRegistryName(), "inventory")); } public static void registerItemRenderer(Item item, int meta, String fileName) { Minecraft.getMinecraft().getRenderItem().getItemModelMesher() .register(item, meta, new ModelResourceLocation((fileName), "inventory")); } } Item Class package com.thatcreepyuncle.logicalTech.item; import java.util.List; import com.thatcreepyuncle.logicalTech.util.ModCreativeTabs; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; public class Circuit extends Item{ public Circuit() { super(); this.setHasSubtypes(true); this.setRegistryName("circuit"); this.setCreativeTab(ModCreativeTabs.GENERAL); this.setUnlocalizedName("circuit"); } @Override public String getUnlocalizedName(ItemStack stack) { String name = EnumCircuit.values()[stack.getItemDamage()].getUnlocalizedName(); return "circuit_" + name; } @Override public void getSubItems(Item itemIn, CreativeTabs tab, List subItems) { for (int i = 0; i < 3; i++) { subItems.add(new ItemStack(itemIn, 1, i)); } } }
-
I have moved all of the methods into my Client Proxy, but the textures still don't show, and there isn't an error. BTW, I did Change it to ModelLoader.setCustomModelResourceLocation.
-
I am confused, but in the code above I put it in the Client Proxy? Am I wrong?
-
That still doesn't work: New Client Proxy Code: package com.thatcreepyuncle.logicalTech.core; import net.minecraft.client.renderer.block.model.ModelBakery; import net.minecraft.util.ResourceLocation; public class ClientProxy extends CommonProxy{ @Override public void init() { loadMetaItems(); ModItems.init(); } @Override public void preInit() { super.preInit(); } @Override public void loadMetaItems() { ModelBakery.registerItemVariants(ModItems.circuit, new ResourceLocation("ltech:toolCircuit"), new ResourceLocation("ltech:redstoneCircuit"), new ResourceLocation("ltech:motherboardCircuit")); ModelBakery.registerItemVariants(ModItems.remote_switch, new ResourceLocation("ltech:remote_switch"), new ResourceLocation("ltech:remote_switch_activated")); } }
-
Currently I am trying to add circuits, however the textures are not showing and I can't find a error in the log. LOG 2016-07-07 18:48:29,080 WARN Unable to instantiate org.fusesource.jansi.WindowsAnsiOutputStream 2016-07-07 18:48:29,083 WARN Unable to instantiate org.fusesource.jansi.WindowsAnsiOutputStream [18:48:29] [main/INFO] [GradleStart]: Extra: [] [18:48:29] [main/INFO] [GradleStart]: Running with arguments: [--userProperties, {}, --assetsDir, C:/Users/Bob/.gradle/caches/minecraft/assets, --assetIndex, 1.9, --accessToken{REDACTED}, --version, 1.9.4, --tweakClass, net.minecraftforge.fml.common.launcher.FMLTweaker, --tweakClass, net.minecraftforge.gradle.tweakers.CoremodTweaker] [18:48:29] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.FMLTweaker [18:48:29] [main/INFO] [LaunchWrapper]: Using primary tweak class name net.minecraftforge.fml.common.launcher.FMLTweaker [18:48:29] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.gradle.tweakers.CoremodTweaker [18:48:29] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLTweaker [18:48:29] [main/INFO] [FML]: Forge Mod Loader version 12.17.0.1976 for Minecraft 1.9.4 loading [18:48:29] [main/INFO] [FML]: Java is Java HotSpot(TM) 64-Bit Server VM, version 1.8.0_65, running on Windows 10:amd64:10.0, installed at C:\Program Files\Java\jdk1.8.0_66\jre [18:48:29] [main/INFO] [FML]: Managed to load a deobfuscated Minecraft name- we are in a deobfuscated environment. Skipping runtime deobfuscation [18:48:29] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.gradle.tweakers.CoremodTweaker [18:48:29] [main/INFO] [GradleStart]: Injecting location in coremod net.minecraftforge.fml.relauncher.FMLCorePlugin [18:48:29] [main/INFO] [GradleStart]: Injecting location in coremod net.minecraftforge.classloading.FMLForgePlugin [18:48:29] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.FMLInjectionAndSortingTweaker [18:48:29] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.FMLDeobfTweaker [18:48:29] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.gradle.tweakers.AccessTransformerTweaker [18:48:29] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLInjectionAndSortingTweaker [18:48:29] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLInjectionAndSortingTweaker [18:48:29] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.relauncher.CoreModManager$FMLPluginWrapper [18:48:29] [main/ERROR] [FML]: The binary patch set is missing. Either you are in a development environment, or things are not going to work! [18:48:31] [main/ERROR] [FML]: FML appears to be missing any signature data. This is not a good thing [18:48:31] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.relauncher.CoreModManager$FMLPluginWrapper [18:48:31] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.FMLDeobfTweaker [18:48:33] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.gradle.tweakers.AccessTransformerTweaker [18:48:33] [main/INFO] [LaunchWrapper]: Loading tweak class name net.minecraftforge.fml.common.launcher.TerminalTweaker [18:48:33] [main/INFO] [LaunchWrapper]: Calling tweak class net.minecraftforge.fml.common.launcher.TerminalTweaker [18:48:33] [main/INFO] [LaunchWrapper]: Launching wrapped minecraft {net.minecraft.client.main.Main} 2016-07-07 18:48:34,174 WARN Unable to instantiate org.fusesource.jansi.WindowsAnsiOutputStream 2016-07-07 18:48:34,214 WARN Unable to instantiate org.fusesource.jansi.WindowsAnsiOutputStream 2016-07-07 18:48:34,218 WARN Unable to instantiate org.fusesource.jansi.WindowsAnsiOutputStream [18:48:34] [Client thread/INFO]: Setting user: Player503 [18:48:39] [Client thread/INFO]: LWJGL Version: 2.9.4 [18:48:41] [Client thread/INFO] [sTDOUT]: [net.minecraftforge.fml.client.SplashProgress:start:202]: ---- Minecraft Crash Report ---- // I'm sorry, Dave. Time: 7/7/16 6:48 PM Description: Loading screen debug info This is just a prompt for computer specs to be printed. THIS IS NOT A ERROR A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- System Details -- Details: Minecraft Version: 1.9.4 Operating System: Windows 10 (amd64) version 10.0 Java Version: 1.8.0_65, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 896680584 bytes (855 MB) / 1038876672 bytes (990 MB) up to 1038876672 bytes (990 MB) JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: Loaded coremods (and transformers): GL info: ' Vendor: 'ATI Technologies Inc.' Version: '4.5.13416 Compatibility Profile Context 15.300.1025.1001' Renderer: 'AMD Radeon R7 200 Series' [18:48:41] [Client thread/INFO] [FML]: MinecraftForge v12.17.0.1976 Initialized [18:48:41] [Client thread/INFO] [FML]: Replaced 232 ore recipes [18:48:41] [Client thread/INFO] [FML]: Found 0 mods from the command line. Injecting into mod discoverer [18:48:41] [Client thread/INFO] [FML]: Searching C:\Users\Bob\Desktop\LogicalTech 1.9.4\run\mods for mods [18:48:41] [Client thread/INFO] [ltech]: Mod ltech is missing the required element 'name'. Substituting ltech [18:48:43] [Client thread/INFO] [FML]: Forge Mod Loader has identified 4 mods to load [18:48:43] [Client thread/INFO] [FML]: Attempting connection with missing mods [mcp, FML, Forge, ltech] at CLIENT [18:48:43] [Client thread/INFO] [FML]: Attempting connection with missing mods [mcp, FML, Forge, ltech] at SERVER [18:48:44] [Client thread/INFO]: Reloading ResourceManager: Default, FMLFileResourcePack:Forge Mod Loader, FMLFileResourcePack:Minecraft Forge, FMLFileResourcePack:ltech [18:48:44] [Client thread/INFO] [FML]: Processing ObjectHolder annotations [18:48:44] [Client thread/INFO] [FML]: Found 418 ObjectHolder annotations [18:48:44] [Client thread/INFO] [FML]: Identifying ItemStackHolder annotations [18:48:44] [Client thread/INFO] [FML]: Found 0 ItemStackHolder annotations [18:48:44] [Client thread/INFO] [FML]: Configured a dormant chunk cache size of 0 [18:48:44] [Forge Version Check/INFO] [ForgeVersionCheck]: [Forge] Starting version check at http://files.minecraftforge.net/maven/net/minecraftforge/forge/promotions_slim.json [18:48:45] [Client thread/INFO] [FML]: Applying holder lookups [18:48:45] [Forge Version Check/INFO] [ForgeVersionCheck]: [Forge] Found status: UP_TO_DATE Target: null [18:48:45] [Client thread/INFO] [FML]: Holder lookups applied [18:48:45] [Client thread/INFO] [FML]: Injecting itemstacks [18:48:45] [Client thread/INFO] [FML]: Itemstack injection complete [18:48:49] [sound Library Loader/INFO]: Starting up SoundSystem... [18:48:49] [Thread-8/INFO]: Initializing LWJGL OpenAL [18:48:49] [Thread-8/INFO]: (The LWJGL binding of OpenAL. For more information, see http://www.lwjgl.org) [18:48:49] [Thread-8/INFO]: OpenAL initialized. [18:48:50] [sound Library Loader/INFO]: Sound engine started [18:48:56] [Client thread/INFO] [FML]: Max texture size: 16384 [18:48:56] [Client thread/INFO]: Created: 16x16 textures-atlas [18:48:58] [Client thread/INFO] [FML]: Injecting itemstacks [18:48:58] [Client thread/INFO] [FML]: Itemstack injection complete [18:48:58] [Client thread/INFO] [FML]: Forge Mod Loader has successfully loaded 4 mods [18:48:58] [Client thread/INFO]: Reloading ResourceManager: Default, FMLFileResourcePack:Forge Mod Loader, FMLFileResourcePack:Minecraft Forge, FMLFileResourcePack:ltech [18:49:02] [Client thread/INFO]: SoundSystem shutting down... [18:49:02] [Client thread/WARN]: Author: Paul Lamb, www.paulscode.com [18:49:02] [sound Library Loader/INFO]: Starting up SoundSystem... [18:49:02] [Thread-10/INFO]: Initializing LWJGL OpenAL [18:49:02] [Thread-10/INFO]: (The LWJGL binding of OpenAL. For more information, see http://www.lwjgl.org) [18:49:02] [Thread-10/INFO]: OpenAL initialized. [18:49:03] [sound Library Loader/INFO]: Sound engine started [18:49:07] [Client thread/INFO] [FML]: Max texture size: 16384 [18:49:08] [Client thread/INFO]: Created: 1024x512 textures-atlas [18:49:10] [Realms Notification Availability checker #1/INFO]: Could not authorize you against Realms server: Invalid session id [18:50:17] [server thread/INFO]: Starting integrated minecraft server version 1.9.4 [18:50:17] [server thread/INFO]: Generating keypair [18:50:17] [server thread/INFO] [FML]: Injecting existing block and item data into this server instance [18:50:17] [server thread/INFO] [FML]: Applying holder lookups [18:50:17] [server thread/INFO] [FML]: Holder lookups applied [18:50:17] [server thread/INFO] [FML]: Loading dimension 0 (Dev World) (net.minecraft.server.integrated.IntegratedServer@3e33e4ba) [18:50:18] [server thread/INFO] [FML]: Loading dimension 1 (Dev World) (net.minecraft.server.integrated.IntegratedServer@3e33e4ba) [18:50:18] [server thread/INFO] [FML]: Loading dimension -1 (Dev World) (net.minecraft.server.integrated.IntegratedServer@3e33e4ba) [18:50:18] [server thread/INFO]: Preparing start region for level 0 [18:50:20] [Netty Local Client IO #0/INFO] [FML]: Server protocol version 2 [18:50:20] [Netty Server IO #1/INFO] [FML]: Client protocol version 2 [18:50:20] [Netty Server IO #1/INFO] [FML]: Client attempting to join with 4 mods : [email protected],[email protected],[email protected],[email protected] [18:50:20] [Netty Local Client IO #0/INFO] [FML]: [Netty Local Client IO #0] Client side modded connection established [18:50:20] [server thread/INFO] [FML]: [server thread] Server side modded connection established [18:50:20] [server thread/INFO]: Player503[local:E:6a2fdb05] logged in with entity id 1253 at (19.42612807298208, -120.6795103751531, -4.886012161662722) [18:50:20] [server thread/INFO]: Player503 joined the game [18:50:21] [server thread/INFO]: Saving and pausing game... [18:50:21] [server thread/INFO]: Saving chunks for level 'Dev World'/Overworld [18:50:21] [server thread/INFO]: Saving chunks for level 'Dev World'/Nether [18:50:21] [server thread/INFO]: Saving chunks for level 'Dev World'/The End [18:50:21] [pool-2-thread-1/WARN]: Couldn't look up profile properties for com.mojang.authlib.GameProfile@3c394e9e[id=6b7bc9c9-e7da-3a47-9bb3-2051e22e10b9,name=Player503,properties={},legacy=false] com.mojang.authlib.exceptions.AuthenticationException: The client has sent too many requests within a certain amount of time at com.mojang.authlib.yggdrasil.YggdrasilAuthenticationService.makeRequest(YggdrasilAuthenticationService.java:65) ~[YggdrasilAuthenticationService.class:?] at com.mojang.authlib.yggdrasil.YggdrasilMinecraftSessionService.fillGameProfile(YggdrasilMinecraftSessionService.java:175) [YggdrasilMinecraftSessionService.class:?] at com.mojang.authlib.yggdrasil.YggdrasilMinecraftSessionService$1.load(YggdrasilMinecraftSessionService.java:59) [YggdrasilMinecraftSessionService$1.class:?] at com.mojang.authlib.yggdrasil.YggdrasilMinecraftSessionService$1.load(YggdrasilMinecraftSessionService.java:56) [YggdrasilMinecraftSessionService$1.class:?] at com.google.common.cache.LocalCache$LoadingValueReference.loadFuture(LocalCache.java:3524) [guava-17.0.jar:?] at com.google.common.cache.LocalCache$Segment.loadSync(LocalCache.java:2317) [guava-17.0.jar:?] at com.google.common.cache.LocalCache$Segment.lockedGetOrLoad(LocalCache.java:2280) [guava-17.0.jar:?] at com.google.common.cache.LocalCache$Segment.get(LocalCache.java:2195) [guava-17.0.jar:?] at com.google.common.cache.LocalCache.get(LocalCache.java:3934) [guava-17.0.jar:?] at com.google.common.cache.LocalCache.getOrLoad(LocalCache.java:3938) [guava-17.0.jar:?] at com.google.common.cache.LocalCache$LocalLoadingCache.get(LocalCache.java:4821) [guava-17.0.jar:?] at com.google.common.cache.LocalCache$LocalLoadingCache.getUnchecked(LocalCache.java:4827) [guava-17.0.jar:?] at com.mojang.authlib.yggdrasil.YggdrasilMinecraftSessionService.fillProfileProperties(YggdrasilMinecraftSessionService.java:165) [YggdrasilMinecraftSessionService.class:?] at net.minecraft.client.Minecraft.getProfileProperties(Minecraft.java:3043) [Minecraft.class:?] at net.minecraft.client.resources.SkinManager$3.run(SkinManager.java:131) [skinManager$3.class:?] at java.util.concurrent.Executors$RunnableAdapter.call(Executors.java:511) [?:1.8.0_65] at java.util.concurrent.FutureTask.run(FutureTask.java:266) [?:1.8.0_65] at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1142) [?:1.8.0_65] at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:617) [?:1.8.0_65] at java.lang.Thread.run(Thread.java:745) [?:1.8.0_65] [18:50:26] [server thread/INFO]: Player503 has just earned the achievement [Taking Inventory] [18:50:26] [Client thread/INFO]: [CHAT] Player503 has just earned the achievement [Taking Inventory] ModItems Class package com.thatcreepyuncle.logicalTech.core; import java.util.ArrayList; import com.thatcreepyuncle.logicalTech.item.Circuit; import com.thatcreepyuncle.logicalTech.item.RemoteSwitch; import net.minecraft.client.Minecraft; import net.minecraft.client.renderer.block.model.ModelResourceLocation; import net.minecraft.item.Item; import net.minecraftforge.fml.common.registry.GameRegistry; public class ModItems { public static ArrayList<Item> items; public static Item circuit; public static Item remote_switch; public static void preInit() { items = new ArrayList<Item>(); circuit = new Circuit(); GameRegistry.register(circuit); remote_switch = new RemoteSwitch(); GameRegistry.register(remote_switch); for (Item item : items) { GameRegistry.register(item); } } public static void init() { registerItemRenderer(circuit, 0, "toolCircuit"); registerItemRenderer(circuit, 1, "redstoneCircuit"); registerItemRenderer(circuit, 2, "motherboardCircuit"); registerItemRenderer(remote_switch, 0, "remote_switch"); registerItemRenderer(remote_switch, 1, "remote_switch_activated"); for (Item item : items) { registerItemRenderer(item); } } public static void registerItemRenderer(Item item) { Minecraft.getMinecraft().getRenderItem().getItemModelMesher() .register(item, 0, new ModelResourceLocation(item.getRegistryName(), "inventory")); } public static void registerItemRenderer(Item item, int meta, String fileName) { Minecraft.getMinecraft().getRenderItem().getItemModelMesher() .register(item, meta, new ModelResourceLocation((fileName), "inventory")); } } Item Class: package com.thatcreepyuncle.logicalTech.item; import java.util.List; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; public class Circuit extends Item{ public Circuit() { super(); this.setHasSubtypes(true); this.setRegistryName("circuit"); this.setCreativeTab(CreativeTabs.REDSTONE); this.setUnlocalizedName("circuit"); } @Override public String getUnlocalizedName(ItemStack stack) { String name = EnumCircuit.values()[stack.getItemDamage()].getUnlocalizedName(); if(name == null){ name = ""; } return "circuit_" + name; } @Override public void getSubItems(Item itemIn, CreativeTabs tab, List subItems) { for (int i = 0; i < 3; i++) { subItems.add(new ItemStack(itemIn, 1, i)); } } } Client Proxy: package com.thatcreepyuncle.logicalTech.core; import net.minecraft.client.renderer.block.model.ModelBakery; import net.minecraft.util.ResourceLocation; public class ClientProxy extends CommonProxy{ @Override public void init() { ModItems.init(); } @Override public void loadMetaItems() { ModelBakery.registerItemVariants(ModItems.circuit, new ResourceLocation("ltech:toolCircuit"), new ResourceLocation("ltech:redstoneCircuit"), new ResourceLocation("ltech:motherboardCircuit")); ModelBakery.registerItemVariants(ModItems.remote_switch, new ResourceLocation("ltech:remote_switch"), new ResourceLocation("ltech:remote_switch_activated")); } }
-
ItemRenderer NullPointerException[SOLVED]
ThatCreepyUncle replied to ThatCreepyUncle's topic in Modder Support
Thanks -
So I am messing around with forge 1.9, and a machine. It will craft circuits in a crafting table kind of thing. However, whenever I create a new ItemStack in the output slot, I get an error. TileEntity Class: package com.thatcreepyuncle.logicalTech.tileEntity; import com.thatcreepyuncle.logicalTech.crafting.CircuitWorkbenchCraftingManager; import io.netty.util.internal.SystemPropertyUtil; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.inventory.ISidedInventory; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.nbt.NBTTagList; import net.minecraft.network.NetworkManager; import net.minecraft.network.Packet; import net.minecraft.network.play.server.SPacketUpdateTileEntity; import net.minecraft.tileentity.TileEntity; import net.minecraft.util.EnumFacing; import net.minecraft.util.ITickable; import net.minecraft.util.text.ITextComponent; import net.minecraft.util.text.TextComponentString; public class TileEntityCircuitWorkbench extends TileEntity implements ITickable, ISidedInventory { private static final int[] slots = new int[] { 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 }; public ItemStack[] inventory = new ItemStack[11]; private String customName = "circuit_workbench"; public CircuitWorkbenchCraftingManager manager; public TileEntityCircuitWorkbench() { manager = new CircuitWorkbenchCraftingManager(this); System.out.println("Google"); } @Override public void update() { manager.update(); } public int getSizeInventory() { return 11; } @Override public ItemStack getStackInSlot(int par1) { return this.inventory[par1]; } @Override public ItemStack decrStackSize(int par1, int par2) { if (this.inventory[par1] != null) { ItemStack var3; if (this.inventory[par1].stackSize <= par2) { var3 = this.inventory[par1]; this.inventory[par1] = null; this.markDirty(); return var3; } var3 = this.inventory[par1].splitStack(par2); if (this.inventory[par1].stackSize == 0) { this.inventory[par1] = null; } this.markDirty(); return var3; } return null; } @Override public ItemStack removeStackFromSlot(int par1) { if (this.inventory[par1] != null) { ItemStack var2 = this.inventory[par1]; this.inventory[par1] = null; return var2; } return null; } @Override public void setInventorySlotContents(int index, ItemStack stack) { this.inventory[index] = stack; if (stack != null && stack.stackSize > this.getInventoryStackLimit()) { stack.stackSize = this.getInventoryStackLimit(); } this.markDirty(); } /** * Reads a tile entity from NBT. */ public void readFromNBT(NBTTagCompound tagCompound) { super.readFromNBT(tagCompound); NBTTagList tagList = (NBTTagList) tagCompound.getTag("Items"); this.inventory = new ItemStack[this.getSizeInventory()]; for (int count = 0; count < tagList.tagCount(); ++count) { NBTTagCompound nbt = (NBTTagCompound) tagList.getCompoundTagAt(count); int slot = nbt.getByte("Slot") & 255; if (slot >= 0 && slot < this.inventory.length) { this.inventory[slot] = ItemStack.loadItemStackFromNBT(nbt); } } if (tagCompound.hasKey("CustomName", ) { this.customName = tagCompound.getString("CustomName"); } inventory[0] = null; } @Override public void writeToNBT(NBTTagCompound par1NBTTagCompound) { super.writeToNBT(par1NBTTagCompound); NBTTagList var2 = new NBTTagList(); for (int var3 = 0; var3 < this.inventory.length; ++var3) { if (this.inventory[var3] != null) { NBTTagCompound var4 = new NBTTagCompound(); var4.setByte("Slot", (byte) var3); this.inventory[var3].writeToNBT(var4); var2.appendTag(var4); } } par1NBTTagCompound.setTag("Items", var2); } @Override public void onDataPacket(NetworkManager net, SPacketUpdateTileEntity pkt) { NBTTagCompound tagCom = pkt.getNbtCompound(); this.readFromNBT(tagCom); } @Override public Packet getDescriptionPacket() { NBTTagCompound tagCom = new NBTTagCompound(); this.writeToNBT(tagCom); return new SPacketUpdateTileEntity(pos, getBlockMetadata(), tagCom); } @Override public int getInventoryStackLimit() { return 64; } @Override public boolean isUseableByPlayer(EntityPlayer par1EntityPlayer) { return this.worldObj.getTileEntity(pos) != this ? false : par1EntityPlayer.getDistanceSq(this.pos.getX() + 0.5D, this.pos.getY() + 0.5D, this.pos.getZ() + 0.5D) <= 64.0D; } @Override public void invalidate() { this.updateContainingBlockInfo(); super.invalidate(); } @Override public boolean isItemValidForSlot(int par1, ItemStack par2ItemStack) { return true; } @Override public String getName() { return this.hasCustomName() ? this.customName : "container.compacter"; } @Override public boolean hasCustomName() { return this.customName != null && this.customName.length() > 0; } @Override public ITextComponent getDisplayName() { return new TextComponentString(getName()); } @Override public void openInventory(EntityPlayer playerIn) { } @Override public void closeInventory(EntityPlayer playerIn) { } @Override public int getField(int id) { return 0; } @Override public void setField(int id, int value) { } @Override public int getFieldCount() { return 0; } @Override public void clear() { for (int i = 0; i < inventory.length; i++) { inventory[i] = null; } } @Override public int[] getSlotsForFace(EnumFacing side) { return slots; } @Override public boolean canInsertItem(int index, ItemStack stack, EnumFacing direction) { return true; } @Override public boolean canExtractItem(int index, ItemStack stack, EnumFacing direction) { return false; } } Crafting Manager: package com.thatcreepyuncle.logicalTech.crafting; import com.thatcreepyuncle.logicalTech.tileEntity.TileEntityCircuitWorkbench; import com.thatcreepyuncle.logicalTech.util.FuelHandler; import net.minecraft.client.Minecraft; import net.minecraft.init.Blocks; import net.minecraft.init.Items; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; public class CircuitWorkbenchCraftingManager { // Blocks to Items! private final Item redstoneBlock = Item.getItemFromBlock(Blocks.redstone_block); private final Item ironBlock = Item.getItemFromBlock(Blocks.iron_block); /* * CRAFTING RECIPES */ private final Item[] toolsCircuitInput = new Item[] { redstoneBlock, Items.golden_pickaxe, redstoneBlock, Items.golden_axe, ironBlock, Items.golden_sword, redstoneBlock, Items.golden_shovel, redstoneBlock, Items.diamond }; /* * END OF CRAFTING RECIPES */ private TileEntityCircuitWorkbench tile; public boolean crafting = false; private Item itemCrafting; public int cookTime = 0; public int fuelTime; public CircuitWorkbenchCraftingManager(TileEntityCircuitWorkbench t) { tile = t; } // 2-11 = Input, 0 = Fuel, 1 = output public void update() { if (!crafting) { checkCraft(); } else { System.out.println(this.cookTime); handleFuel(); handleCraft(); } for (int i = 0; i < this.tile.inventory.length; i++) { if (this.tile.inventory[i] != null) if (this.tile.inventory[i].stackSize <= 0) { this.tile.inventory[i] = null; } } } private void handleFuel() { if(fuelTime <= 0 && tile.inventory[1] != null){ if(FuelHandler.isFuel(this.tile.inventory[1].getItem())){ fuelTime = FuelHandler.calcFuel(this.tile.inventory[1]); } } } private void startCraft(Item[] recipe, ItemStack[] input){ crafting = true; for (int i = 0; i < input.length; i++) { input[i].stackSize--; } cookTime = 1000; } private void handleCraft() { if(fuelTime > 0){ if(cookTime > 0){ cookTime--; fuelTime--; }else{ craft(); crafting = false; } } } private void checkCraft() { Item[] input = new Item[9]; ItemStack[] inputStacks = new ItemStack[9]; for (int i = 0; i < 9; i++) { if (tile.inventory[i + 2] == null) { break; } inputStacks[i] = tile.inventory[i + 2]; input[i] = tile.inventory[i + 2].getItem(); } if (canCraft(input, this.toolsCircuitInput)) { startCraft(this.toolsCircuitInput, inputStacks); } } private void craft() { System.out.println("Crafting:"); if (this.tile.inventory[0] == null) { System.out.println("Inventory[0]"); this.tile.inventory[0] = new ItemStack(itemCrafting); } else { System.out.println("Googles"); this.tile.inventory[0].stackSize++; } } private boolean canCraft(Item[] input, Item[] recipe) { if (tile.inventory[0] != null) { if (tile.inventory[0].getItem() == recipe[9] && tile.inventory[0].stackSize < 64) { for (int i = 0; i < input.length; i++) { if (input[i] != recipe[i]) { return false; } } return true; } } else { for (int i = 0; i < input.length; i++) { if (input[i] != recipe[i]) { return false; } } return true; } return false; } } Crash Log: A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Stacktrace: at net.minecraft.client.renderer.RenderItem.renderItemOverlayIntoGUI(RenderItem.java:428) at net.minecraft.client.gui.inventory.GuiContainer.drawSlot(GuiContainer.java:319) at net.minecraft.client.gui.inventory.GuiContainer.drawScreen(GuiContainer.java:118) at net.minecraftforge.client.ForgeHooksClient.drawScreen(ForgeHooksClient.java:353) -- Screen render details -- Details: Screen name: com.thatcreepyuncle.logicalTech.gui.CircuitWorkbenchGui Mouse location: Scaled: (403, 214). Absolute: (806, 51) Screen size: Scaled: (427, 240). Absolute: (854, 480). Scale factor of 2 -- Affected level -- Details: Level name: MpServer All players: 1 total; [EntityPlayerSP['Player962'/0, l='MpServer', x=8.20, y=4.00, z=4.30]] Chunk stats: MultiplayerChunkCache: 289, 289 Level seed: 0 Level generator: ID 01 - flat, ver 0. Features enabled: false Level generator options: Level spawn location: World: (8,4,, Chunk: (at 8,0,8 in 0,0; contains blocks 0,0,0 to 15,255,15), Region: (0,0; contains chunks 0,0 to 31,31, blocks 0,0,0 to 511,255,511) Level time: 122022 game time, 1304 day time Level dimension: 0 Level storage version: 0x00000 - Unknown? Level weather: Rain time: 0 (now: false), thunder time: 0 (now: false) Level game mode: Game mode: creative (ID 1). Hardcore: false. Cheats: false Forced entities: 1 total; [EntityPlayerSP['Player962'/0, l='MpServer', x=8.20, y=4.00, z=4.30]] Retry entities: 0 total; [] Server brand: fml,forge Server type: Integrated singleplayer server Stacktrace: at net.minecraft.client.multiplayer.WorldClient.addWorldInfoToCrashReport(WorldClient.java:445) at net.minecraft.client.Minecraft.addGraphicsAndWorldToCrashReport(Minecraft.java:2766) at net.minecraft.client.Minecraft.run(Minecraft.java:422) at net.minecraft.client.main.Main.main(Main.java:118) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:497) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:497) at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) at GradleStart.main(GradleStart.java:26) -- System Details -- Details: Minecraft Version: 1.9 Operating System: Windows 10 (amd64) version 10.0 Java Version: 1.8.0_65, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 551814400 bytes (526 MB) / 1038876672 bytes (990 MB) up to 1038876672 bytes (990 MB) JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: MCP 9.23 Powered by Forge 12.16.1.1887 4 mods loaded, 4 mods active States: 'U' = Unloaded 'L' = Loaded 'C' = Constructed 'H' = Pre-initialized 'I' = Initialized 'J' = Post-initialized 'A' = Available 'D' = Disabled 'E' = Errored UCHIJAAAA mcp{9.19} [Minecraft Coder Pack] (minecraft.jar) UCHIJAAAA FML{8.0.99.99} [Forge Mod Loader] (forgeSrc-1.9-12.16.1.1887.jar) UCHIJAAAA Forge{12.16.1.1887} [Minecraft Forge] (forgeSrc-1.9-12.16.1.1887.jar) UCHIJAAAA ltech{1.0} [ltech] (bin) Loaded coremods (and transformers): GL info: ' Vendor: 'ATI Technologies Inc.' Version: '4.5.13416 Compatibility Profile Context 15.300.1025.1001' Renderer: 'AMD Radeon R7 200 Series' Launched Version: 1.9 LWJGL: 2.9.4 OpenGL: AMD Radeon R7 200 Series GL version 4.5.13416 Compatibility Profile Context 15.300.1025.1001, ATI Technologies Inc. GL Caps: Using GL 1.3 multitexturing. Using GL 1.3 texture combiners. Using framebuffer objects because OpenGL 3.0 is supported and separate blending is supported. Shaders are available because OpenGL 2.1 is supported. VBOs are available because OpenGL 1.5 is supported. Using VBOs: No Is Modded: Definitely; Client brand changed to 'fml,forge' Type: Client (map_client.txt) Resource Packs: Current Language: English (US) Profiler Position: N/A (disabled) CPU: 4x AMD FX(tm)-4300 Quad-Core Processor [10:45:17] [Client thread/INFO] [sTDOUT]: [net.minecraft.init.Bootstrap:printToSYSOUT:646]: #@!@# Game crashed! Crash report saved to: #@!@# C:\Users\Noah\Desktop\Computer Mod 1.9\run\.\crash-reports\crash-2016-07-05_10.45.17-client.txt AL lib: (EE) alc_cleanup: 1 device not closed Java HotSpot(TM) 64-Bit Server VM warning: Using incremental CMS is deprecated and will likely be removed in a future release