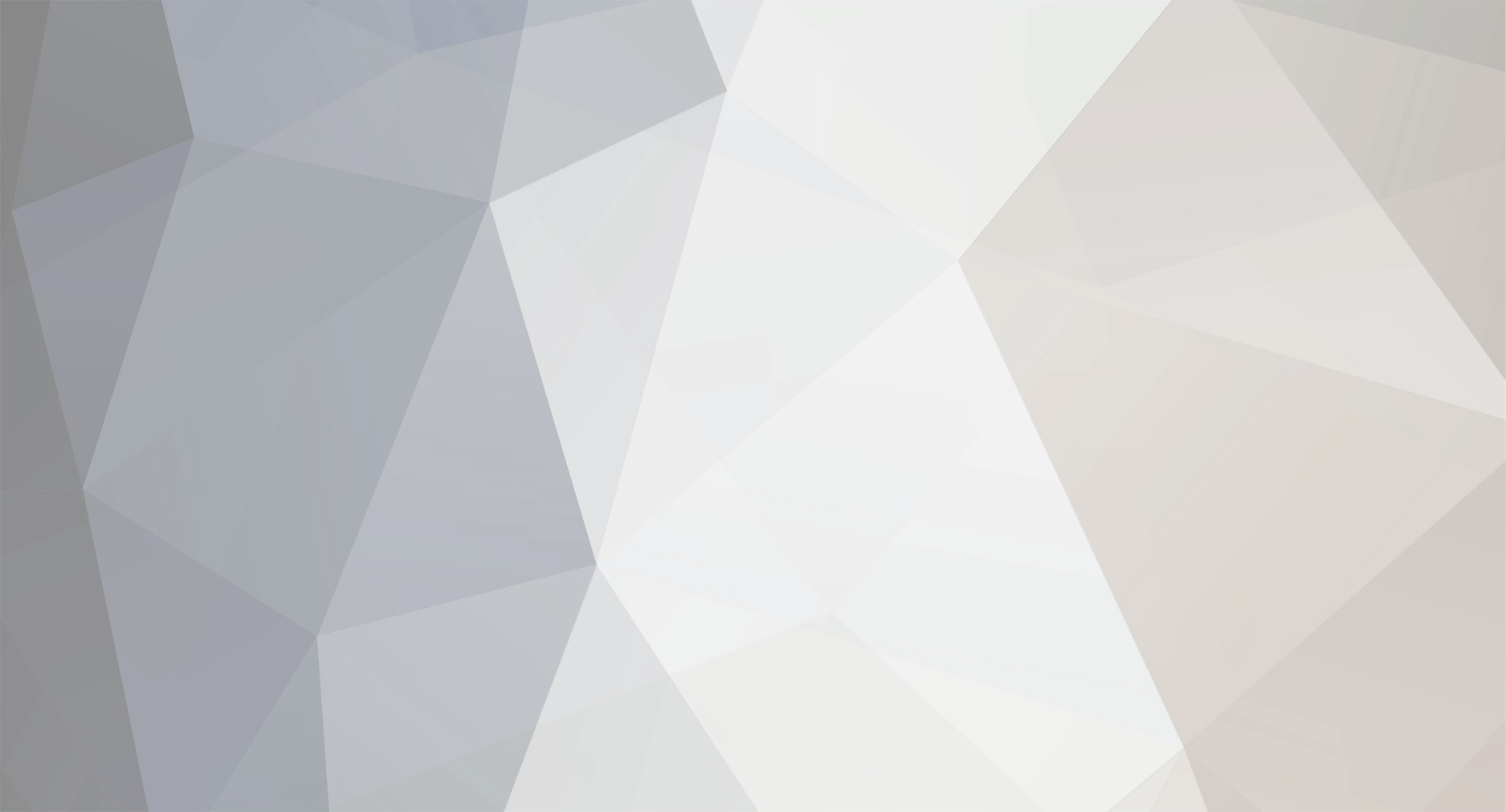
DreadKyller
Members-
Posts
27 -
Joined
-
Last visited
Converted
-
Gender
Male
-
Personal Text
bleh... blah...
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
DreadKyller's Achievements

Tree Puncher (2/8)
2
Reputation
-
checks the 6 adjacent sides of the cable in question and finds any blocks that are of your cable type. If it's of your cable type then get the tile entity at that location via world.getTileEntity(x, y, z) and add it to a list.
-
The most common are NBT and damage values. Upon using your wand set it to 0 damage, then wit the onUpdate method increase the damage value by 1. The damage bar would be an indicator to the time. When the damage is maxed, the tool is ready again. If you must use the damage for something else, then you can store an integer in the NBT of the item stack.
-
Yes, this should work, however another option commonly used is as follows: 1: When a cable is placed, check if there are cabled next to it. 2: If no cables are next to it, create a custom TileEntity there. This will be the master tile entity for that system. Don't proceed farther down the logic. 3: If there were cables attached, get the tile entity at that location and find it's master. 4: Create a tile entity at the location of the placed cable, and set it's master to the master of the cables that are attached. 5: If the cables have different masters, we need to combine them into one system. Choose one of the systems and set all the When a cable is broken, you need to separate the cables into separate systems: 1: Iterate through the cables attached to each of the cabled next to the one that was broken, check if they are still connected to their master. If not, set them as the master of their own system, otherwise return and stop doing the next logic. 2: Iterate through the connected cables that were made into their own system and set their master to the new one. To check is a block is connected to the system, get the master of the cable next to the machine and see if that tile entity has certain data. The tile entity would store the following: TileEntity master boolean isMaster int powerInSystem etc... The code would be similar to the following: public void addCable(World w, int x, int y, int z) { // Create your tile entity here and add it to the world ... // Rest of code List<TileEntityPowerSystem> systems= getConnectedWires(w, x, y, z); if(systems.size() == 0) { tileEntity.setIsMaster(true); return; } TileEntityPowerSystem master = systems.get(0).getMaster();// Select the first system as the master system tileEntity.setMaster(master) for(TileEntityPowerSystem system : systems) { if(system != master) { system.getMaster().setMaster(master);// Make the master tile entities of the other systems no longer masters // And set their master to the new master. } } } public int getPower(World w, int x, int y, int z) { TileEntity te = w.getTileEntityAt(x, y, z); if(te instanceof TileEntityPowerSystem) { return ((TileEntityPowerSystem)te).getMaster().getPowerInSystem(); } return 0; } etc... This method requires using more, smaller, tile entities than one single one which holds all the information. In return it makes al operations faster, instead of having the cylce through or iterate through all the connected blocks, you can use stored data to access directly the tile entity that has been put in charge of storing the main data for the system. public TileEntityPowerSystem getMaster() { if(!this.master.isMaster) { this.master = this.master.getMaster();// If the blocks previous master was merged with another system, update it now } return this.master; } I don't have the development environment up atm, but this method probably isn't completely safe with chunk loading/unloading. You may want to store positions for the master instead of the actual tile entity to avoid issues, and maybe have the tile entities store a n instance of a object called System (or whatever) that stores the actual data instead of the tile entity, so that it can be access from anywhere, even if the master is unloaded.
-
I'm going to assume you wish to have this structure variable sized? If so here's a few steps of the process. Note that this is for explaination purposes, there are more efficient ways of doing parts of this, but as it's a task for a lesson you can try to figure some of that out Do something similar to these when your blocks are placed: 1: Identify the corners of your structure: 1a: Since this seems to be class project related, I won't give exact instructions 1b: determine if the block was placed on a wall of floor/ceiling (using blocks next to it) 1c: Depending, iterate through the blocks negative and positive to find the corner blocks. 1d: For easy later, it's easiest to make the corners the absolute negative corner and the absolute positive corner. 2: Now that you have the corners, check the border blocks: 2a: Start at the negative corner: 2b: For each axis iterate and check the blocks the ensure they're the proper type 2c: Do the same with the positive corner. 3: Check the walls of the cuboid for your type: 3a:you can get a wall by taking 2 of the axis from one point and iterating towards the values of the other point, but always keeping the remaining axis equal to the value of the starting point. 3b: You can also (although less efficient as it cycles through each block) cycle through each block, a block is located on the wall (or floor/ceiling) if any of the coordinates are equal on any axis to the values of either corner, all blocks in the middle will have 0 matching locations (the x will not be equal to either of the point's x location, same for y and z) 4: If these conditions are met, create the tile entity, store the two corners inside the tile entity. 5: On the tile entity update you can perform your operations within the two corners (be careful with how intensive your operation is or how often you set the tile to be ticked, you can cause a lot of lag). 6: You may have issues if your greenhouse crosses multiple chunks, if the chunk the tile entity is in isn't loaded, but the rest ofthe greenhouse is, the greenhouse will not function. If you need it to function regardless you can setup a master-slave system with the tile entities. Create a tile entity on each of the 4 corners on the bottom (or top if you wish) of your greenhouse. Set one as the master, another one as the secondary, another as the third, and the last as the fourth. When these tick they will first check if the tile entity one higher on the master-slave ladder is loaded, if so it will tick that instead, this would continue until it reaches the highest ranked loaded tile entity. On the highest ranked tile entity check if it has already ticked within the last while (however long you have between ticks) to prevent the entity ticking too often. At least one of the corners will be loaded any time any of the greenhouse is loaded (unless the greenhouse is like 32 or something chunks wide, which in that case that's a major problem). 7: You can also choose to, if you wish to make it easier at the cost of some slight memory, you can create a tile entity per chunk in that greenhouse that doesn't tick. Upon reaking a block of your type, you may iterate through the list of tile entites in that chunk, find that one and you may store on that tile entity the locationns of the 4 master-slave tile entities. Based on these locations you can determine with higher efficiency whether your block you broke was part of the greenouse (without having to do the stages in 1 again). If it was, destroy the tile entities, your greenhouse has been deconstructed.
-
[1.8] Craft a certain item with any sword/pickaxe/etc
DreadKyller replied to SuperSaltyGamer's topic in Modder Support
Another thing to add is don't expect every modder to use the standards, even though you should extend ItemSword there are definitely modders out there that do not. You should use what Ernio posted above, but note that if you want your mod to be completely compatible with certain mods, you may need to add specific support for the mods that don't use standard practices. -
[1.8] Craft a certain item with any sword/pickaxe/etc
DreadKyller replied to SuperSaltyGamer's topic in Modder Support
Can you post the stacktrace so we can see what line is causing the NullPointerException? I'm going to assume however that this line is going to cause problems: for (int i = 0; i < 8; i++) { if (inv.getStackInSlot(i).getUnlocalizedName().contains("sword")) { ... } } inv.getStackInSlot(i) may return null there, and then you're calling null.getUnlocalizedName(). This may be causing your exception. you should add another null check there. -
Those methods were added in 1.8 and are not available in 1.7. 1.8 introduced a new system of block models, for which those methods were created. The tutorial you're using (if any) is from 1.8. The RenderItem type you're trying to get the ModelMesher from is the physical renderer for Item Entities. The class that getRenderItem() returns in 1.8 doesn't exist either in 1.7.10. In 1.8 the block model and the textures are set inside the JSON model. pre 1.8 textures were registered via the methods setBlockTextureName and registerBlockIcons within the block class.
-
Ok, if you're checking the block in the isprovidingWeakPower, then you can just call the notifyBlocksOfNeighborChange within the onNeighborChange. public void onNeighborBlockChange(World world, int x, int y, int z, Block block) { world.notifyBlocksOfNeighborChange(x, y, z, this); }
-
I edited my last reply, but didn't finish before you viewed.
-
You should execute that whenever you change the signal that you are outputting, so wherever that is being changed, add that line. In the case of what you're doing, you'd put it in the onNeighborBlockChange. onNeighborBlockChange: 1) Check if there is a block connected to the front face 2) Set the metadata of the block depending on that result 3) call the notifyBlocksOfNeighborChange isProvidingWeakPower: 1) return a boolean based off the metadata that you set in the onNeighborBlockChange 2) You can get that metadata using the IBlockAccess.getBlockMetadata 3) The first 3 integers in the isProvidingWeakPower are the X, Y and Z coords. An example of the metadata could be: 0 ) North - No power 1 ) South - No power 2 ) East - No power 3 ) West - No power 4 ) Up - No power 5 ) Down - No power 6 ) North - Power 7 ) South - Power 8 ) East - Power 9 ) West - Power 10 ) Up - Power 11 ) Down - Power You can get direction (0-5) using meta % 6 You can get power using meta > 5 If you only want North, South, East and Wesk, just make it 0-7, use meta % 4 and meta > 3
-
world.notifyBlocksOfNeighborChange(int x, int y, int z, Block b) requires the location of the block that changed, it automatically calls the function world.nofityBlockOfNeighborChange(int x, int y, int z, Block b) for all 6 connected blocks. Btw, as of this message, congrats on 64 posts, you're now a full stack!
-
onNeighborBlockChange is for when a nearby block changes, it is not used to update other blocks when the block is placed. If you look at the redstone torch code you'll see: world.notifyBlocksOfNeighborChange You may need to execute this for the blocks that your redstone device is connecting to to cause those blocks to update and detect that the block is providing them power.
-
You appear to be registering the block to the GameRegistry inside the constructor for the block. One question I'd like to ask is in your main class are you creating a new instance of the block? If not then the call to the registry is never being executed.
-
[1.8] Can't find any schematic to Java Converters
DreadKyller replied to ShadowBeastGod's topic in Modder Support
What type of schematic are you referring to? If you're talking about the types of schematics created by MCEdit and WorldEdit and various other external programs? If so it's not really "converted". You can read up on the format here. You may have to create your own parser for the format as not many public tools appear to exist for it. However to aid you, you can find some code that could make it easier to understand here You may also be interested in checking out a related thread If you're referring to converting a schematic to hardcoded java, that's a bit more tricky, although there are some resources that seem to have done it. These are old, but the code should still work with slight modifications, and should at least give you something to work with, -
I didn't say anything about the parsing of the JSON being the thing that slows it down, why are you putting words in my mouth? I stated a fact that the same exact model in two different versions of Minecraft, giving massive differences in performance, I didn't blame the JSON parsing, but something isn't as fast when dealing with complex models. I understand that the JSOn is only parsed at the creation of the object and then cached for use later when being rendered, and I havn't modded much with 1.8 so I don't understand exactly how the new system works, as in how the data is physically stored (my guess would be some form of list storing a primitive type that defines the corners texture and vertex UV's of each box) and how that data is then used when rendering (I'm guessing iterating through the list and calling the GL11.glVertex3f and GL11.glTexCoords2f with the data on the current cuboid). Those are only my guesses, having never actually viewed the code. In my experience, every time I've used the 1.8 object format the performance was far worse than the same model in 1.7, you can discuss the efficiency of the method as much as is possible, it won't change the fact that I personally have had issues with it. I said this conversation is getting off topic, I will not reply to this again because it's becoming a stupid argument over different peoples experience with a feature.