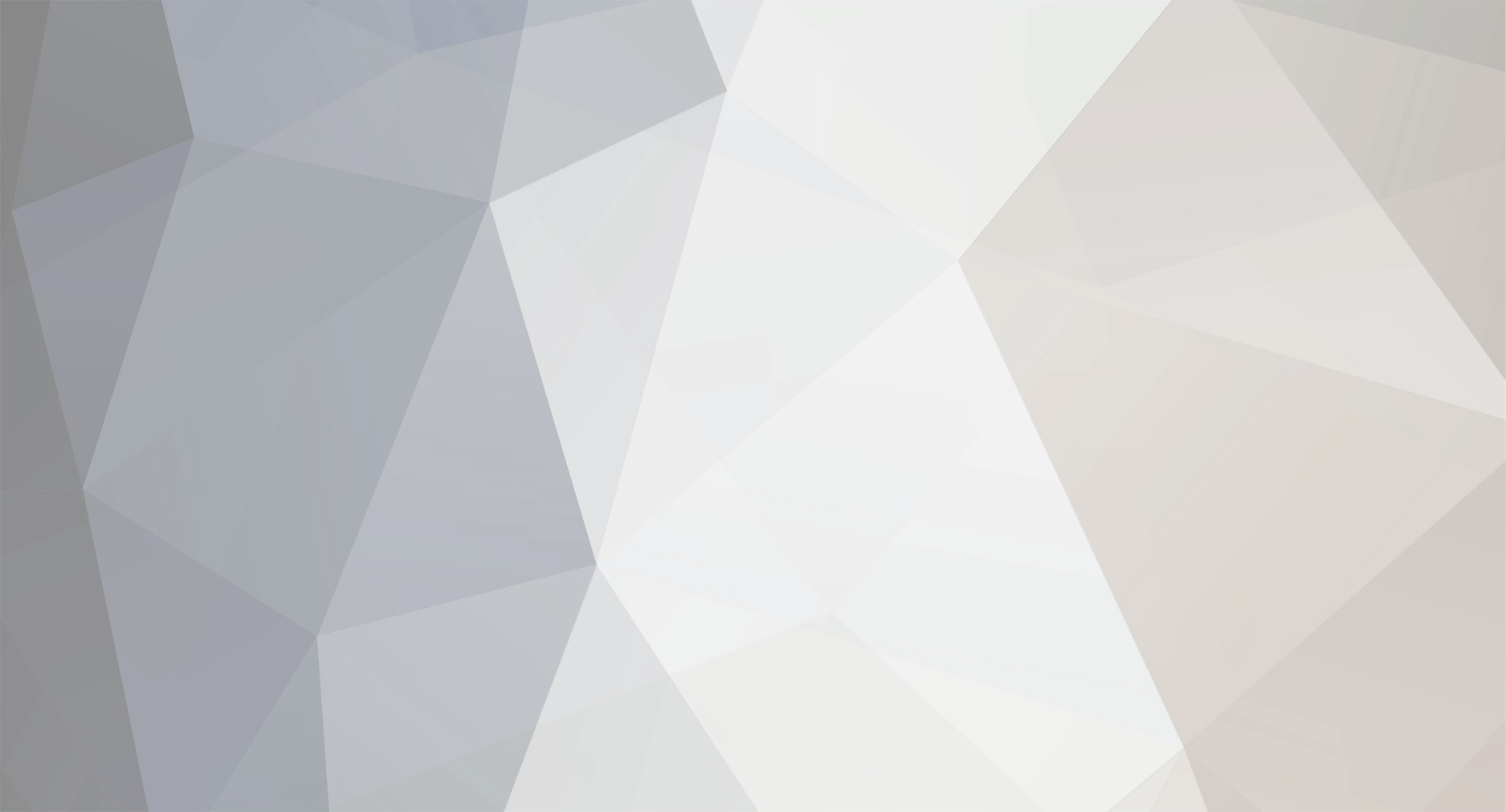
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
Crash on world load, java out of bounds exception
TheGreyGhost replied to Deco's topic in Modder Support
Hi java.lang.ArrayIndexOutOfBoundsException: 117 potionTypes[this.potionID] so potionID is out of range (117 but the maximum allowed is 31) The only place that potionID is set is during construction of PotionEffect. If it crashed after world load, this might means that PotionEffect is not reloading from the NBT properly? public static PotionEffect readCustomPotionEffectFromNBT(NBTTagCompound par0NBTTagCompound) { byte b0 = par0NBTTagCompound.getByte("Id"); byte b1 = par0NBTTagCompound.getByte("Amplifier"); int i = par0NBTTagCompound.getInteger("Duration"); boolean flag = par0NBTTagCompound.getBoolean("Ambient"); return new PotionEffect(b0, i, b1, flag); } Could you post your code that does the application of the PotionEffect? You could also try: Add a statement to onUpdate and put a breakpoint on it, then wait for the breakpoint to trigger and step your way back out to see why potionID was set to 117. public boolean onUpdate(EntityLivingBase par1EntityLivingBase) { if (this.duration > 0) { if (this.potionID >= 32) { this.potionID = this.potionID; // ADD A BREAKPOINT HERE } else if (Potion.potionTypes[this.potionID].isReady(this.duration, this.amplifier)) -TGG -
Hmmm Maybe you could add a custom smelting recipe for your item, so that when you put it into the furnace, it turns into a different "unstable" item that explodes as soon as it's created (i.e. as soon as any code tries to call any of its methods - onCreated might be suitable?). So perhaps you could (1) override MyItem.onCreated() to set a flag in your "TriggerExplosion" class (2) add a TickHandler which checks the TriggerExplosion class every tick (3) if the TriggerExplosion flag is set, it looks near the player for any TileEntityFurnaces, finds the one with the explosive item in it, then blows it up -TGG
-
Are There Tutorials For This Type of Thing?
TheGreyGhost replied to Gamerguy0811's topic in Modder Support
Hi The best advice I can give is to learn by doing. Start really simple, watch a basic tutorial, get someone else's sample code, run it, understand how it works, change one or two small things and see what happens, then slowly start introducing stuff of your own. After a while it all starts to make sense. Realistically you should expect it to take weeks to months before you get reasonably good at it. That's part of the fun of programming I reckon- you start off rubbish and slowly learn and discover things, until one day you realise "hey I'm not too bad at this after all". -TGG -
Hi I suspect you might be able to intercept Packets by creating your own MyNetClientHandler extends NetClientHandler, ithen overwriting EntityClientPlayerMP.sendQueue with MyNetClientHandler. http://greyminecraftcoder.blogspot.com.au/2013/10/client-side-class-linkage-map.html Duplicating the NetClientHandler object would be the hard part, I'm not sure it's possible using .clone() and many of the member fields are private so you couldn't copy them directly. You might be able to store a reference to the original NetClientHandler inside your MyNetClientHandler, and then override all the functions with accessors to the NetClientHandler eg MyNetClientHandler. public void processReadPackets() { this.netClientHandler.processReadPackets() } however you would then need to make sure that you keep the public fields in MyNetClientHandler up to date. Either way I think it would be pretty messy, and I hear that 1.7 completely updates packets anyway, so you might want to look for other ways perhaps... -TGG
-
Hi Maybe I've misunderstood what you said here - how can you tell which client is making the sound if you only have one set of speakers? I'd suggest you use two separate clients (on separate PCs) just to make sure there isn't something funny going on. Also, with two PCs you can insert a breakpoint on one and then step through the code to see which method makes the sound play on the other client. Perplexing... -TGG
-
Hi I've got a sneaking suspicion that onInventoryChanged is not called for all changes to the inventory. To be honest, I wouldn't worry about your original idea being "inefficient", Java is pretty quick and comparing a few slots for changes takes basically no time at all. I'd suggest you have another go with it because I reckon it should work fine. -TGG
-
Probably not Possible - Getting a world reference
TheGreyGhost replied to Draco18s's topic in Modder Support
Hi Hmmm that sounds pretty ambitious. I had a quick look through NetClientHandler.handleRespawn() and it looks like you would have a lot of work to do in order to set up the second world properly, and I'm not sure that you could actually happily co-exist two WorldClients at the same time. You might be able to get the effect you want if you actually teleport the player to the new dimension but "freeze" the game from updating or somehow prevent the player from appearing in the world or interacting with it, until they "commit" to the teleport. -TGG -
Hi Are you sure that SoundManager.playSoundFX tells the Server to play a sound to other clients? I don't see any evidence of that in the code, there are no Packet or NetworkHandler imports in the class, and I don't recall seeing any Client->Server packets related to sound generation. http://greyminecraftcoder.blogspot.com.au/2013/10/packets-from-client-to-server.html -TGG
-
Block texures not loading after mod installation
TheGreyGhost replied to Stormageddon's topic in Modder Support
Hi This might help give more diagnostic info: Change the vanilla source code to add two println statements as below. Then copy us your logs for (1) startup during development environment (2) startup when packaged into a zip -TGG FolderResourcePack protected boolean makeFullPath(String par1Str) { System.out.println("FolderResourcePack.makeFullPath:" + this.basePath + " , " + par1Str); // add this return (new File(this.basePath, par1Str)).isFile(); } FileResourcePack protected boolean makeFullPath(String par1Str) { System.out.println("FileResourcePack.makeFullPath:" + this.basePath + " , " + par1Str); // add this return (new File(this.basePath, par1Str)).isFile(); } -
Hi To honest, I really doubt there is any way that the server can tell if the client is cheat-modded. All the server can see from the client is packets, and cheaters can change the client code to give whatever the server expects to see. MD5 won't help you because the client can send you whatever MD5 it likes. -TGG
-
Hi This might help: Change the vanilla source code to add two println statements as below. Then start up minecraft and copy us your error log. -TGG FolderResourcePack protected boolean makeFullPath(String par1Str) { System.out.println("FolderResourcePack.makeFullPath:" + this.basePath + " , " + par1Str); // add this return (new File(this.basePath, par1Str)).isFile(); } FileResourcePack protected boolean makeFullPath(String par1Str) { System.out.println("FileResourcePack.makeFullPath:" + this.basePath + " , " + par1Str); // add this return (new File(this.basePath, par1Str)).isFile(); }
-
Making a block orient towards the play on block place
TheGreyGhost replied to DJFergy's topic in Modder Support
Hi This link will give the basic idea http://www.minecraftforge.net/forum/index.php/topic,13626.0.html You just need to extend the if block to include top and bottom as well. How to convert your yaw and pitch to a face? There are a couple of ways - if you want it to face based on where the player is looking only, then using the yaw and pitch will be fine. I'd suggest: (1) if pitch is more than 45 degrees above horizontal, you're looking at the top face (2) if pitch is more than 45 degrees below horizontal, you're looking at the bottom face (3) if the pitch is within 45 degrees of the horizontal, then the yaw tells you which of the four side faces you're looking at. You might also consider just using the PistonBase.determineOrientation method, if the vanilla method for placing a piston is good enough for what you want. -TGG -
Probably not Possible - Getting a world reference
TheGreyGhost replied to Draco18s's topic in Modder Support
Hi This might help http://greyminecraftcoder.blogspot.com.au/2013/10/client-side-class-linkage-map.html http://greyminecraftcoder.blogspot.com.au/2013/10/server-side-class-linkage-map.html WorldClient is probably easy WorldServer might be harder if you can't tell what world the player is in -TGG -
Hi Robin4002 is right, you can also use setTextureName instead of registerIcons if it is more convenient. Both methods will work the same if you have the same texture for all faces. I'm guessing your error message "Using missing texture, unable to load:" should have changed now to 2013-11-06 13:23:00 [sEVERE] [Minecraft-Client] Using missing texture, unable to load: minecraft:textures/blocks/StrongStone.png If forge still can't find your textures, try this link: http://www.minecraftforge.net/forum/index.php?topic=11963.0 Also http://www.minecraftforge.net/forum/index.php/topic,13355.msg69061.html#msg69061 (Apparently - when you're using Eclipse (which I'm not) you should put it into the SOURCE folder, wherever that is) -TGG
-
Hi I think the basic concept should work fine. Depending on how you are storing the slot contents, the problem might be here if(this.lastSlots != this.currentSlots) i.e. this statement compares two objects, so these might be different objects even if the contents are identical alternatively, perhaps you are not updating lastSlots or currentSlots properly? Have you tried inserting a breakpoint and inspecting them, or logging their contents to stdout? -TGG
-
ha, nicely spotted, I was scratching my head over that one :-) -TGG
-
Hi Curious! Do you know how to use breakpoints? I'd suggest putting a breakpoint into your onEaten and looking at the stack trace to see which thread it's in and who is calling it each time. -TGG
-
Hi You might find these links interesting http://greyminecraftcoder.blogspot.com.au/2013/10/the-most-important-minecraft-classes.html http://greyminecraftcoder.blogspot.com.au/2013/10/client-side-class-linkage-map.html http://greyminecraftcoder.blogspot.com.au/2013/10/server-side-class-linkage-map.html http://greyminecraftcoder.blogspot.com.au/2013/10/client-server-communication-using.html -TGG
-
Hi What you've set is the name of the picture file used for rendering the block. You also need to set a unique name for the block, using setUnlocalizedName("myname") i.e. public StrongStone(int id, Material Material) { super(id, Material); setUnlocalizedName("StrongStone"); } You can tell this from the error message: 2013-11-06 13:23:00 [sEVERE] [Minecraft-Client] Using missing texture, unable to load: minecraft:textures/blocks/MISSING_ICON_TILE_500_StrongStone.png i.e. MISSING_ICON_TILE_500_ -TGG
-
[SOLVED] How does a metadata block drop metadata items?
TheGreyGhost replied to SkylordJoel's topic in Modder Support
no worries, glad it helped :-) -TGG -
[Unsolved] Crash from IItemRenderer for my custom model[1.6]
TheGreyGhost replied to larsgerrits's topic in Modder Support
Hi Ah, I meant your TileEntityRenderer. I would suggest to copy your rendering code out of the TileEntityRenderer and put it into your IItemRenderer class. Or use a simpler icon for the item. -TGG -
Get rid of the protection enchanting calculation
TheGreyGhost replied to zerozhou's topic in Modder Support
Hi Sort of - I mean for example @ForgeSubscribe public void myLivingHurt(LivingHurtEvent event) { DamageSource damageSource = event.source; float amount = event.ammount; EntityLivingBase entityLivingBase = event.entityLiving; float damage = amount; // par2 = ForgeHooks.onLivingHurt(this, par1DamageSource, par2); // if (par2 <= 0) return; // par2 = this.applyArmorCalculations(par1DamageSource, par2); // par2 = this.applyPotionDamageCalculations(par1DamageSource, par2); float f1 = damage; damage = Math.max(damage - entityLivingBase.getAbsorbtion(), 0.0F); entityLivingBase.func_110149_m(entityLivingBase.getAbsorbtion() - (f1 - damage)); if (damage != 0.0F) { float f2 = entityLivingBase.getHealth(); entityLivingBase.setEntityHealth(f2 - damage); entityLivingBase.func_110142_aN().func_94547_a(par1DamageSource, f2, damage); entityLivingBase.func_110149_m(this.getAbsorbtion() - damage); } return -1; } -TGG -
[SOLVED] How does a metadata block drop metadata items?
TheGreyGhost replied to SkylordJoel's topic in Modder Support
Hi If your ItemID is always the same, and only the metadata changes, you can use damageDropped and idDropped as we described. If you want to vary the ItemID as well as the damage value, you could consider overriding Block.getBlockDropped /** * This returns a complete list of items dropped from this block. * * @param world The current world * @param x X Position * @param y Y Position * @param z Z Position * @param metadata Current metadata * @param fortune Breakers fortune level * @return A ArrayList containing all items this block drops */ public ArrayList<ItemStack> getBlockDropped(World world, int x, int y, int z, int metadata, int fortune) { ArrayList<ItemStack> ret = new ArrayList<ItemStack>(); int count = quantityDropped(metadata, fortune, world.rand); for(int i = 0; i < count; i++) { int id = idDropped(metadata, world.rand, fortune); if (id > 0) { ret.add(new ItemStack(id, 1, damageDropped(metadata))); } } return ret; } -TGG -
Hi Your function never returns true because if(mc.thePlayer.getHeldItem() == new ItemStack(MyMod.Knife)) is never true. getHeldItem is a different object to the new ItemStack even if they are identical. try instead if(mc.thePlayer.getHeldItem().itemID == MyMod.Knife.itemID)) -TGG
-
[Unsolved] Crash from IItemRenderer for my custom model[1.6]
TheGreyGhost replied to larsgerrits's topic in Modder Support
Hi I would suggest to copy your rendering code out of the TileEntity and put it into your IItemRenderer class. Or use a simpler icon for the item. -TGG