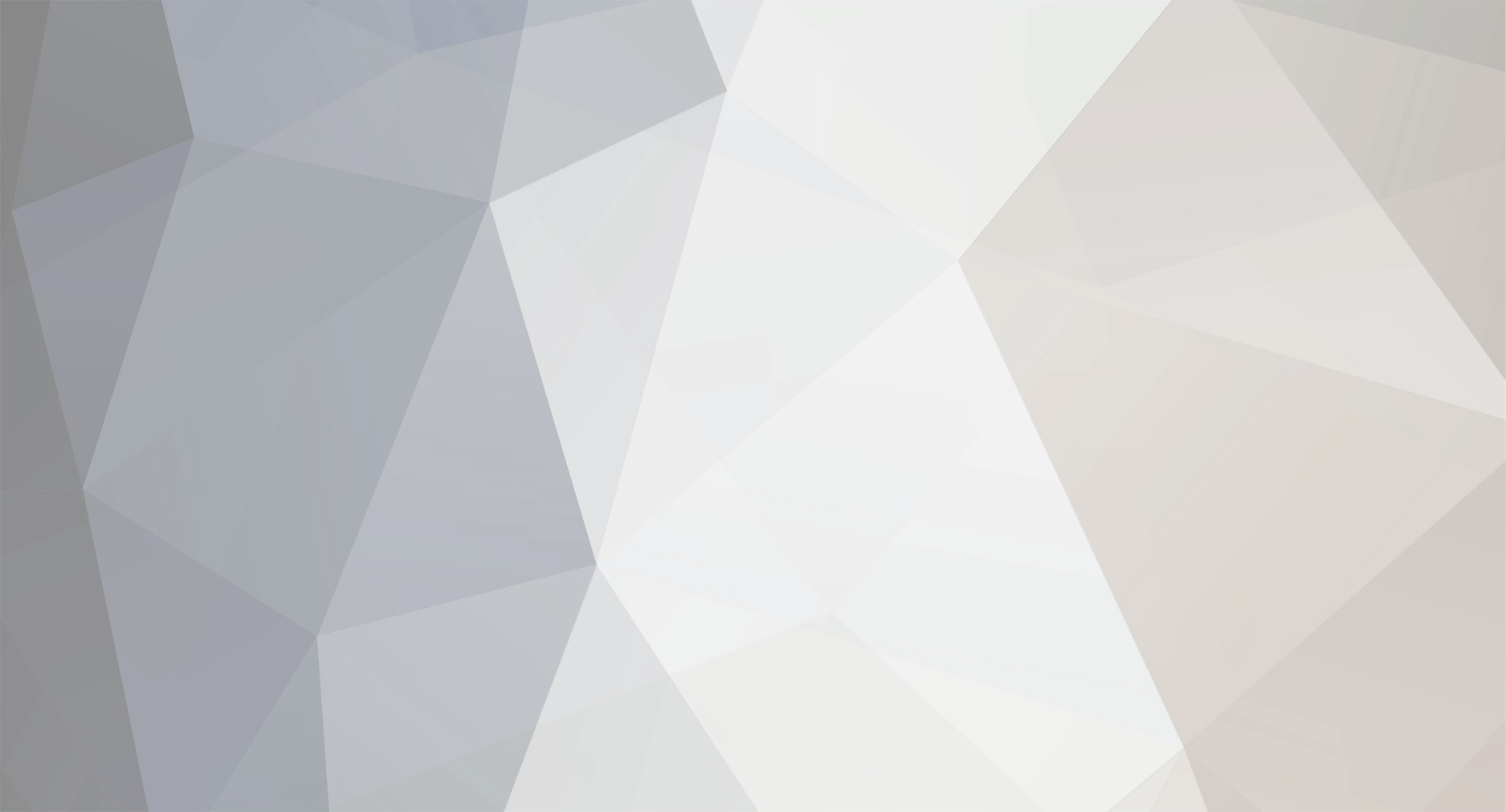
Jiro7
Members-
Posts
31 -
Joined
-
Last visited
Everything posted by Jiro7
-
Not sure if this is what you meant by reflection, as I've never done that yet (I know basic Java, but not that much), but this is what I did and got a crash. In my main.java: @EventHandler public static void init(FMLInitializationEvent event){ Collection<VillagerProfession> profs = ForgeRegistries.VILLAGER_PROFESSIONS.getValuesCollection(); for (VillagerProfession pr : profs){ if(pr.getSkin().toString().equals("minecraft:textures/entity/villager/librarian.png")){ pr.getCareer(0).getTrades(0).remove(1); pr.getCareer(0).getTrades(3).remove(0); pr.getCareer(0).getTrades(4).remove(0); pr.getCareer(0).getTrades(0).add(1, new ListEnchantedBookNew()); pr.getCareer(0).getTrades(3).add(0, new ListEnchantedBookNew()); pr.getCareer(0).getTrades(4).add(0, new ListEnchantedBookNew()); } } } The new class: public class ListEnchantedBookNew implements EntityVillager.ITradeList { @Override public void addMerchantRecipe(IMerchant merchant, MerchantRecipeList recipeList, Random random) { Enchantment enchantment = (Enchantment)Enchantment.REGISTRY.getRandomObject(random); while (!(enchantment instanceof EnchantmentSlimy || enchantment instanceof EnchantmentShieldBoost || enchantment instanceof EnchantmentAbsorb)){ enchantment = (Enchantment)Enchantment.REGISTRY.getRandomObject(random); } int i = MathHelper.getInt(random, enchantment.getMinLevel(), enchantment.getMaxLevel()); ItemStack itemstack = ItemEnchantedBook.getEnchantedItemStack(new EnchantmentData(enchantment, i)); int j = 2 + random.nextInt(5 + i * 10) + 3 * i; if (enchantment.isTreasureEnchantment()) { j *= 2; } if (j > 64) { j = 64; } recipeList.add(new MerchantRecipe(new ItemStack(Items.BOOK), new ItemStack(Items.EMERALD, j), itemstack)); } } And the crash:
-
Is there really no easy way to do this without having to do the PR, though? Like, creating a custom villager class that extends from the original one but overrides the trade for books method, and then replace villagers with the new ones. The only problem I see is that ListEnchantedBookForEmeralds is a static class and not a method, but I feel like there must be a way. I literally just want to replace Enchantment enchantment = (Enchantment)Enchantment.REGISTRY.getRandomObject(random); by Enchantment enchantment = (Enchantment)Enchantment.REGISTRY.getRandomObject(random); while (enchantment instanceof EnchantmentMyEnchant){ enchantment = (Enchantment)Enchantment.REGISTRY.getRandomObject(random); } in ListEnchantedBookForEmeralds
-
How can I see the source code, though? I went ahead and try to add a PR, but the code in MinecraftForge/patches/minecraft/net/minecraft/enchantment/EntityVillager.java.patch is way different than the one I see at my own eclipse when I go to net.minecraft.enchantment.EntityVillager.java so I don't know exactly where to edit ListEnchantedBookForEmeralds
-
Thank you for the answer. I will try to do the PR, it seems much easier.
-
So... is it not possible?
-
My mod adds new enchantments, and I gave them special ways to be obtained (drop from certain mob). I want to disable ANY other way to obtain the enchanted book. Making them "treasure" enchantments fixed the enchanting table part, but even treasure enchantments can still be found in some chest loot and in villager trades. I can edit the loot tables for the chest part, but how do I disable those specific trades? Even if I disabled village generation, players could still just cure a zombie villager and breed them, and I don't want to remove villagers. I just want to remove some specific trades.
-
Title. I have an enchantment that gives player 30 seconds of resistance for the cost of 30 durability, which is activated when sneaking + right click the item. The ability works fine, except that the effect never ends and stays at 0 seconds permanently. The only way to fix it is closing the world and entering again. Additional note: the sound effect also doesn't seem to work @SubscribeEvent public static void sneakBoost(InputUpdateEvent event){ if (event.getMovementInput().sneak){ EntityPlayer player = event.getEntityPlayer(); if (player.getActiveItemStack().getItem().isShield(player.getActiveItemStack(), player)){ if (!player.isPotionActive(MobEffects.RESISTANCE)){ player.getHeldItemOffhand().damageItem(30, player); player.addPotionEffect(new PotionEffect(MobEffects.RESISTANCE, 600, 0)); player.world.playSound(null, player.posX, player.posY, player.posZ, SoundEvents.BLOCK_SHULKER_BOX_CLOSE, SoundCategory.NEUTRAL, 2.0F, 1.0F); } } } }
-
Nvm, found it, this is the solution for anyone else wondering: public static void sneakBoost(InputUpdateEvent event){ if (event.getMovementInput().sneak){ // rest of the code
-
I want to add a new enchantment that activates an ability when sneaking (player pressing shift). I tried looking all types of PlayerEvent and there doesn't seem to be anyone related to sneaking. Any help?
-
I'm making a mod that adds enchantments to shield and I want to make one that increases the distance that deflected arrows travel. My plan is to use my custom shield class (that extends from ItemShield) to override the function that implements the arrow deflecting functionality, however I can't find it. All I could find related to "blocking" is this code: this.addPropertyOverride(new ResourceLocation("blocking"), new IItemPropertyGetter() { @SideOnly(Side.CLIENT) public float apply(ItemStack stack, @Nullable World worldIn, @Nullable EntityLivingBase entityIn) { return entityIn != null && entityIn.isHandActive() && entityIn.getActiveItemStack() == stack ? 1.0F : 0.0F; } }); BlockDispenser.DISPENSE_BEHAVIOR_REGISTRY.putObject(this, ItemArmor.DISPENSER_BEHAVIOR); However I don't know how to deal with this. Any ideas?
-
How would you do this in 1.12.2? GameRegistry.addSubstitutionAlias method doesn't exist anymore
-
I found out that I need to use GameRegistry.addSubstitutionAlias, but Eclipse doesn't seem to recognize that method, is it outdated??? Is there a new way to do this? Please help All this just to change a 0 by a 1. And no, I don't want to make a new shield item, I want this to work for the vanilla one, so people can get the mod in started worlds and get their enchantments in their already crafted shields.
-
Looking at the code it seems like the issue is that Item class has a method called getItemEnchantability which returns 0, except for classes that override it like bow, tools, armor... but not ItemShield. So my question is, how can I modify the ItemShield class to make shields enchantable? I already have the enchantments added and working with /enchant command. I just need them to be available at enchanting table, which should hopefully be fixed by overriding getItemEnchantability for ItemShield to return 1.
-
Managed to fix this by simply putting public static final EnumEnchantmentType SHIELDS = EnumHelper.addEnchantmentType("shields", (item)->(item instanceof ItemShield)); before public static final Enchantment HEAVYWEIGHT = new EnchantmentHeavyweight(); public static final Enchantment BERSERK = new EnchantmentBerserk(); public static final Enchantment GUARD = new EnchantmentGuard(); public static final Enchantment DEFLECTION = new EnchantmentDeflection(); public static final Enchantment ABSORB = new EnchantmentAbsorb(); public static final Enchantment SHIELD_BOOST = new EnchantmentShieldBoost(); public static final Enchantment SLIMY = new EnchantmentSlimy(); public static final Enchantment TEST = new EnchantmentTest(); Hope it helps others in the same situation!
-
So I'm trying to make a mod that adds enchantments to shields. I haven't added any effects to them yet, but I wanted to test if I could get them to show up in a shield when putting it inside an enchanting table, but they don't. I created a new EnumEnchantmentType called SHIELDS but it doesn't seem to work, I can't get the enchantments ingame. I know this is the case because I created a Test enchantment that, instead of being Shield enchantment type, is "Breakable" enchantment type (which is a vanilla enchantment type) and it's the only one that works. The error: [13:32:03] [Server thread/WARN] [minecraft/CommandHandler]: Couldn't process command: enchant @p shieldenchants:shieldboost java.lang.NullPointerException: null at net.minecraft.item.Item.canApplyAtEnchantingTable(Item.java:1167) ~[Item.class:?] at net.minecraft.enchantment.Enchantment.canApplyAtEnchantingTable(Enchantment.java:226) ~[Enchantment.class:?] at net.minecraft.enchantment.Enchantment.canApply(Enchantment.java:190) ~[Enchantment.class:?] at net.minecraft.command.CommandEnchant.execute(CommandEnchant.java:76) ~[CommandEnchant.class:?] at net.minecraft.command.CommandHandler.tryExecute(CommandHandler.java:126) [CommandHandler.class:?] at net.minecraft.command.CommandHandler.executeCommand(CommandHandler.java:98) [CommandHandler.class:?] at net.minecraft.network.NetHandlerPlayServer.handleSlashCommand(NetHandlerPlayServer.java:1003) [NetHandlerPlayServer.class:?] at net.minecraft.network.NetHandlerPlayServer.processChatMessage(NetHandlerPlayServer.java:979) [NetHandlerPlayServer.class:?] at net.minecraft.network.play.client.CPacketChatMessage.processPacket(CPacketChatMessage.java:47) [CPacketChatMessage.class:?] at net.minecraft.network.play.client.CPacketChatMessage.processPacket(CPacketChatMessage.java:8) [CPacketChatMessage.class:?] at net.minecraft.network.PacketThreadUtil$1.run(PacketThreadUtil.java:21) [PacketThreadUtil$1.class:?] at java.util.concurrent.Executors$RunnableAdapter.call(Unknown Source) [?:1.8.0_191] at java.util.concurrent.FutureTask.run(Unknown Source) [?:1.8.0_191] at net.minecraft.util.Util.runTask(Util.java:53) [Util.class:?] at net.minecraft.server.MinecraftServer.updateTimeLightAndEntities(MinecraftServer.java:798) [MinecraftServer.class:?] at net.minecraft.server.MinecraftServer.tick(MinecraftServer.java:743) [MinecraftServer.class:?] at net.minecraft.server.integrated.IntegratedServer.tick(IntegratedServer.java:192) [IntegratedServer.class:?] at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:592) [MinecraftServer.class:?] at java.lang.Thread.run(Unknown Source) [?:1.8.0_191] [13:32:03] [main/INFO] [minecraft/GuiNewChat]: [CHAT] An unknown error occurred while attempting to perform this command This is the code: The ModEnchantments class: package jiro.shieldenchantments.init; import jiro.shieldenchantments.enchantment.EnchantmentAbsorb; import jiro.shieldenchantments.enchantment.EnchantmentBerserk; import jiro.shieldenchantments.enchantment.EnchantmentDeflection; import jiro.shieldenchantments.enchantment.EnchantmentGuard; import jiro.shieldenchantments.enchantment.EnchantmentHeavyweight; import jiro.shieldenchantments.enchantment.EnchantmentShieldBoost; import jiro.shieldenchantments.enchantment.EnchantmentSlimy; import jiro.shieldenchantments.enchantment.EnchantmentTest; import net.minecraft.enchantment.Enchantment; import net.minecraft.enchantment.EnumEnchantmentType; import net.minecraft.item.ItemShield; import net.minecraftforge.common.util.EnumHelper; import net.minecraftforge.event.RegistryEvent.Register; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.common.eventhandler.SubscribeEvent; import jiro.shieldenchantments.util.Reference; @Mod.EventBusSubscriber(modid = Reference.MOD_ID) public class ModEnchantments { public static final Enchantment HEAVYWEIGHT = new EnchantmentHeavyweight(); public static final Enchantment BERSERK = new EnchantmentBerserk(); public static final Enchantment GUARD = new EnchantmentGuard(); public static final Enchantment DEFLECTION = new EnchantmentDeflection(); public static final Enchantment ABSORB = new EnchantmentAbsorb(); public static final Enchantment SHIELD_BOOST = new EnchantmentShieldBoost(); public static final Enchantment SLIMY = new EnchantmentSlimy(); public static final Enchantment TEST = new EnchantmentTest(); public static final EnumEnchantmentType SHIELDS = EnumHelper.addEnchantmentType("shields", (item)->(item instanceof ItemShield)); @SubscribeEvent public static void registerEnchantments(Register<Enchantment> event) { event.getRegistry().registerAll(HEAVYWEIGHT, BERSERK, GUARD, DEFLECTION, ABSORB, SHIELD_BOOST, SLIMY, TEST); } } One of the new enchantments class (they are all the same): package jiro.shieldenchantments.enchantment; import jiro.shieldenchantments.init.ModEnchantments; import net.minecraft.enchantment.Enchantment; import net.minecraft.enchantment.Enchantment.Rarity; import net.minecraft.inventory.EntityEquipmentSlot; public class EnchantmentSlimy extends Enchantment { public EnchantmentSlimy(){ super(Rarity.COMMON, ModEnchantments.SHIELDS, new EntityEquipmentSlot[] {EntityEquipmentSlot.MAINHAND, EntityEquipmentSlot.OFFHAND}); this.setRegistryName("slimy"); this.setName("slimy"); } @Override public int getMaxLevel() { return 4; } } The Main class: package jiro.shieldenchantments; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.common.Mod.EventHandler; import net.minecraftforge.fml.common.Mod.Instance; import net.minecraftforge.fml.common.SidedProxy; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; import jiro.shieldenchantments.proxy.CommonProxy; import jiro.shieldenchantments.util.Reference; @Mod(modid = Reference.MOD_ID, name = Reference.NAME, version = Reference.VERSION) public class Main { @Instance public static Main instance; @SidedProxy(clientSide = Reference.CLIENT_PROXY_CLASS, serverSide = Reference.COMMON_PROXY_CLASS) public static CommonProxy proxy; @EventHandler public static void PreInit(FMLPreInitializationEvent event){ } @EventHandler public static void init(FMLInitializationEvent event){ } @EventHandler public static void PostInit(FMLPostInitializationEvent event){ } } What am I doing wrong?
-
[1.12.2] Changing the ingredients of a vanilla crafting recipe
Jiro7 replied to Jiro7's topic in Modder Support
I know I should be using something like if (r.getRegistryName() == ???) But I don't know how the vanilla recipe is called -
[1.12.2] Changing the ingredients of a vanilla crafting recipe
Jiro7 replied to Jiro7's topic in Modder Support
How can I do that? Is there any method for that? -
So I want to replace the recipes of furnace and stone tools and make them require a new, more expensive material I created. I managed to remove the recipes by replacing them with a dummy recipe, using a code like this: public static void removeRecipes() { ForgeRegistry<IRecipe> recipeRegistry = (ForgeRegistry<IRecipe>)ForgeRegistries.RECIPES; @SuppressWarnings("deprecation") ArrayList<IRecipe> recipes = Lists.newArrayList(recipeRegistry.getValues()); for (IRecipe r : recipes) { ItemStack output = r.getRecipeOutput(); if (output.getItem() == Item.getItemFromBlock(Blocks.FURNACE)) { recipeRegistry.remove(r.getRegistryName()); recipeRegistry.register(DummyRecipe.from(r)); } } } I have also created my own furnaceJ.json file with the new recipe, however this recipe seems to also be overriden by the dummy recipe. I read that the solution is to register my own recipe instead of the dummy one in this line: recipeRegistry.register(DummyRecipe.from(r)); But... how can I do it? My recipe is saved in a .json file, not in a .java file like the Dummy recipe. I know the solution is probably very simple but I just can't see it.
-
Oh, that was simpler than I thought, thank you.
-
Thanks, I managed to make it drop an item. However, there is only one value for drop chances, how can I give each drop on the list its own probability? So for example I want it to drop between 1-3 iron nuggets and a 10% chance to drop an iron ingot. Is it possible?
-
I've been searching about this but all the answers seem to be outdated. I want to change the drops of vanilla blocks. For example, I want Iron Ores to drop a custom item I made instead of the block itself. I also want to change the amount of lapis dropped. I tried handling the BlockEvent.HarvestDropsEvent event, however it doesn't seem to have any method to change the drops nor drop amounts, just to change the drop chances. Any help?
-
Thank you all for the replies, it finally worked! In case anyone else has this same problem and finds this topic, this is what I did: I created this class: public class OreDisabler { @SubscribeEvent public static void vanillaGenerationAttempt(GenerateMinable event){ if(event.getType().equals(EventType.IRON)){ event.setResult(Result.DENY); } } } And change IRON with whatever ore you want to disable. Also make sure to import the right EventType, the one from GenerateMinable. Then added this: MinecraftForge.ORE_GEN_BUS.register(OreDisabler.class); inside this method of my main mod class: @EventHandler public void init(FMLInitializationEvent event) { ModRecipes.init(); MinecraftForge.ORE_GEN_BUS.register(OreDisabler.class); } Hopefully I can save someone from having the same confusion as I had!
-
[1.12.2] Random damage for sword every time it's crafted
Jiro7 replied to Jiro7's topic in Modder Support
I also tried that, but the original attributes all get removed, but I just want to add additional damage, while keeping the original one and the original attack speed. This is what I tried: @Override public void onCreated(ItemStack stack, World worldIn, EntityPlayer playerIn){ bonusDamage = rand.nextInt(3) + 9; stack.addAttributeModifier(SharedMonsterAttributes.ATTACK_DAMAGE.getName(), new AttributeModifier("Weapon modifier", bonusDamage, 0), EntityEquipmentSlot.MAINHAND); } It says "add" but it doesn't really add, it replaces the old ones, which I don't want. -
Hello, so I'm trying to make a new material with tools that get random bonus attributes when crafted. I'm starting with the sword, which can get a bonus 1-3 damage when crafted. My problem is that, whenever I craft them, the damage of all the previous swords changes too. This is my code: public class ToolSword extends ItemSword implements IHasModel{ private Random rand; private int bonusDamage; public ToolSword(String name, ToolMaterial material){ super(material); setUnlocalizedName(name); setRegistryName(name); setCreativeTab(CreativeTabs.COMBAT); rand = new Random(); bonusDamage = 0; ModItems.ITEMS.add(this); } @Override public void registerModels() { Main.proxy.registerItemRenderer(this, 0, "inventory"); } @Override public void onCreated(ItemStack stack, World worldIn, EntityPlayer playerIn){ bonusDamage = rand.nextInt(3) + 1; } @Override public Multimap<String, AttributeModifier> getAttributeModifiers(EntityEquipmentSlot slot, ItemStack stack) { final Multimap<String, AttributeModifier> modifiers = super.getAttributeModifiers(slot, stack); if (slot == EntityEquipmentSlot.MAINHAND) { replaceModifier(modifiers, SharedMonsterAttributes.ATTACK_DAMAGE, ATTACK_DAMAGE_MODIFIER, bonusDamage); } return modifiers; } private void replaceModifier(Multimap<String, AttributeModifier> modifierMultimap, IAttribute attribute, UUID id, double amount) { // Get the modifiers for the specified attribute final Collection<AttributeModifier> modifiers = modifierMultimap.get(attribute.getName()); // Find the modifier with the specified ID, if any final Optional<AttributeModifier> modifierOptional = modifiers.stream().filter(attributeModifier -> attributeModifier.getID().equals(id)).findFirst(); if (modifierOptional.isPresent()) { // If it exists, final AttributeModifier modifier = modifierOptional.get(); modifiers.remove(modifier); // Remove it modifiers.add(new AttributeModifier(modifier.getID(), modifier.getName(), modifier.getAmount() + amount, modifier.getOperation())); // Add the new modifier } } } I did try using stack.addAttributeModifier which kept each item with its unique value. However, it completely replaces the old damage and attack speed values and the Sharpness enchantment no longer has effect on it, so I don't like using this command because I still want Sharpness to increase its damage even more. Any help?