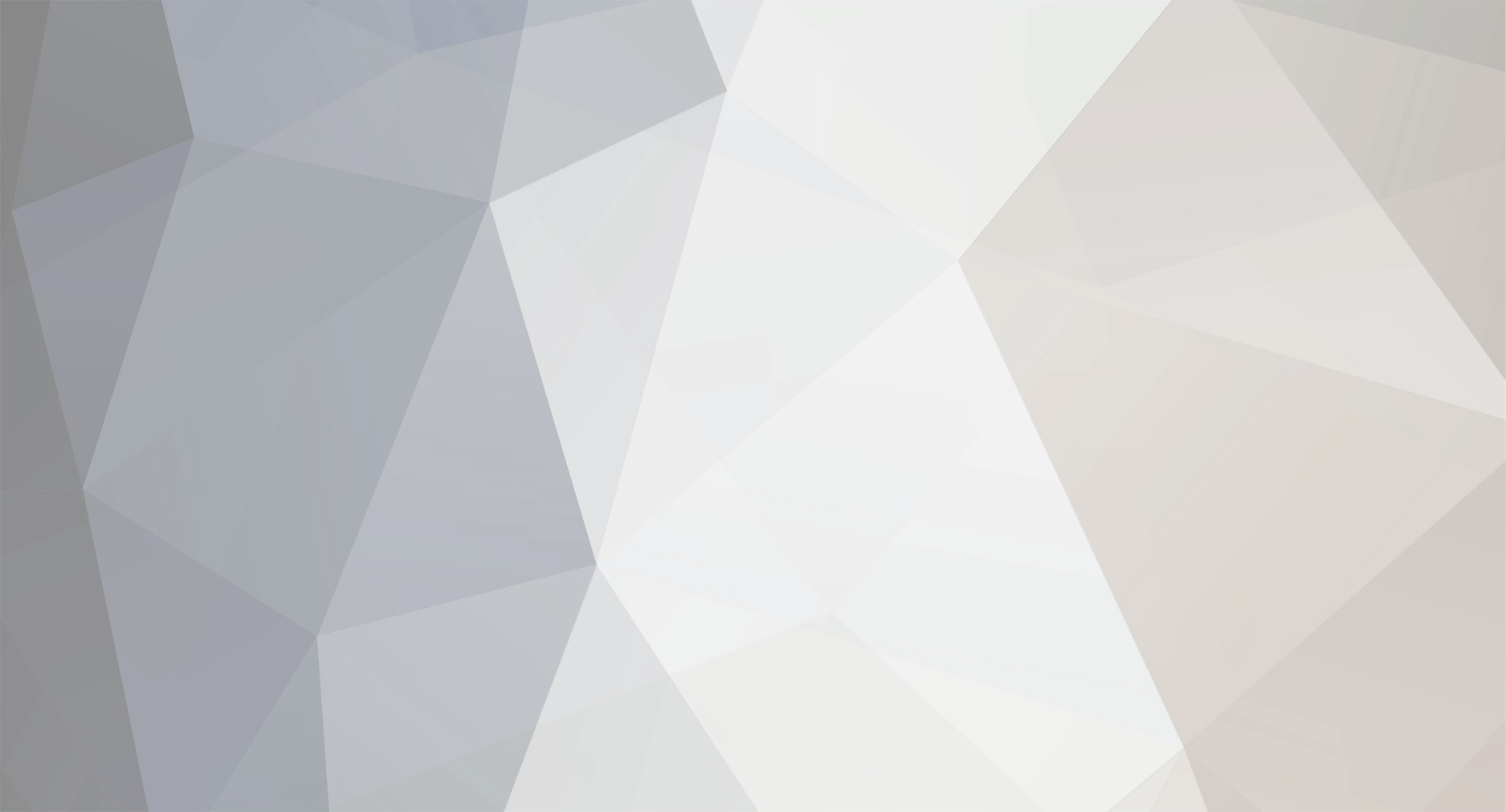
iWasHere
Members-
Posts
24 -
Joined
-
Last visited
Everything posted by iWasHere
-
[1.12] Using an image generated at startup as a block's texture
iWasHere replied to iWasHere's topic in Modder Support
It's not that if they want to change it. It's placed there because I pull block textures from BlockStates which can only be done after texture stitching. The files are stored there so that I can load them in the next time the game is launched. -
[1.12] Using an image generated at startup as a block's texture
iWasHere replied to iWasHere's topic in Modder Support
Thank you for the input, guys. Because the texture is an outside resource (it's located in the config folder), I have no idea how to load it in, as it isn't in my mod's assets directory. I went with DynamicTexture but I really don't think I should be. I was able to load the dummy model, but I'm unsure of how to actually bake it. This is my TextureStitchEvent. It reads in the image fine, I /think/ it's being registered to the TextureMap, but I still get a missing texture error . @SubscribeEvent(priority = EventPriority.LOW) public static void preStitch(TextureStitchEvent.Pre event) { try { BufferedImage image = ImageIO.read(new File("config/compax/cache/minecraft-dirt_x1.png")); ResourceLocation location = Minecraft.getMinecraft().getTextureManager().getDynamicTextureLocation("compax:dirt_x1", new DynamicTexture(image)); TextureAtlasSprite sprite = event.getMap().registerSprite(location); event.getMap().setTextureEntry(sprite); Compax.logger.info("Added this: " + sprite.toString()); } catch (IOException e) { Compax.logger.error("Error reading in texture:\n" + e.getLocalizedMessage()); } } The missing texture error. It's probably the lack of the resource manager? [17:01:07] [Client thread/ERROR] [FML.TEXTURE_ERRORS]: DOMAIN dynamic/compax [17:01:07] [Client thread/ERROR] [FML.TEXTURE_ERRORS]: -------------------------------------------------- [17:01:07] [Client thread/ERROR] [FML.TEXTURE_ERRORS]: domain dynamic/compax is missing 1 texture [17:01:07] [Client thread/ERROR] [FML.TEXTURE_ERRORS]: domain dynamic/compax is missing a resource manager - it is probably a side-effect of automatic texture processing [17:01:07] [Client thread/ERROR] [FML.TEXTURE_ERRORS]: ------------------------- [17:01:07] [Client thread/ERROR] [FML.TEXTURE_ERRORS]: The missing resources for domain dynamic/compax are: [17:01:07] [Client thread/ERROR] [FML.TEXTURE_ERRORS]: textures/dirt_x1_1.png Here's my ModelBakeEvent, runs without error. I'm not sure how to use the texture I added to the TextureMap when retexturing the model here (instead I'm using a texture I have included within my mod's assets folder for the time being). In addition, I have no idea how to actually bake the model. @SubscribeEvent public static void modelBake(ModelBakeEvent event) { Compax.logger.info("Loading models"); try { IModel cube = ModelLoaderRegistry.getModel(new ModelResourceLocation(Compax.MODID + ":dummy")); ImmutableMap.Builder<String, String> texMap = new ImmutableMap.Builder<>(); texMap.put("all", "compax:block/compact_x2"); IModel newCube = cube.retexture(texMap.build()); } catch (Exception e) { Compax.logger.error("Couldn't load models:\n" + e.getLocalizedMessage()); } } A two layer block model might be easier, but I still need to generate these models and apply the textures to them anyways. Sorry about the wall of text, I figured I might as well give as much information as I can. Thank you for your help! -
Hiya! I'm trying to accomplish a few tasks at startup, and anything related to it that I can find was only relevant in 1.7.10. I'm trying to accomplish the following: Grab a block's texture (This works) Put an overlay on the texture (This works, I get a BufferedImage that I can do whatever with. I was only able to do this after the Blockstates have been registered, currently the modified textures are just dumped into a folder under /config/mod. Since this is after TextureStitchEvent, the textures themselves won't exist until after the user launches the game to generate them for the first time, but this isn't too much of a concern to me.) Add the texture to the TextureMap (No idea how to accomplish this) Add a new simple cube model with this new texture on all sides, and have a Block use it Now, the largest issue here is that the blocks that I'm taking the textures of aren't static; the blocks are pulled from a config so the user may select any block they desire the mod to work with. I can't get past adding the new texture to the TextureMap, and I can't find anything at all about adding new models outside of JSON (which doesn't allow them to be generated like I need them to be). I've discovered DynamicTexture, but based on what I've seen I probably don't want to be using that for textures that remain static after startup. Any advice would be appreciated, and thank you for reading. ❤️
-
Ah, thank you!
-
I'm intending to place the key name on a tooltip, I'm not too sure if that's the best way to go about this.
-
What would the best way to get the key for a given action be? For example, I want to get the current bound key for sneak (as a string). Thank you in advance!
-
[1.11.2] Models for block variants won't load?
iWasHere replied to iWasHere's topic in Modder Support
You're a wizard. That worked perfect! Thank you! -
Hi, I'm super tired and having an issue with getting my block variants to have the correct model while displaying in the inventory/hand. They render as a large cube with the missing texture. Here is my ModBlocks class, where the blocks are registered, along with the models. Something is obviously wrong here, as the models aren't being loaded properly for some reason. public class ModBlocks { public static BlockGeodeStone geodeStone; public static ItemBlockMetadata itemblockGeodeStone; public static void register(){ geodeStone = new BlockGeodeStone(BlockNames.GEODE_STONE); itemblockGeodeStone = new ItemBlockMetadata(geodeStone); itemblockGeodeStone.setRegistryName(BlockNames.GEODE_STONE); GameRegistry.register(geodeStone); GameRegistry.register(itemblockGeodeStone); } @SideOnly(Side.CLIENT) public static void preInitRegisterRenderers(){ for (int i = 0; i < BlockNames.GEODE_STONE_VARIANTS.length; i++) { ModelResourceLocation resourceLocation = new ModelResourceLocation(BeyondMod.MODID+":"+BlockNames.GEODE_STONE_VARIANTS[i], "inventory"); ModelLoader.setCustomModelResourceLocation(itemblockGeodeStone, i, resourceLocation); } } @SideOnly(Side.CLIENT) public static void registerRenderers(){ for (int i = 0; i < BlockNames.GEODE_STONE_VARIANTS.length; i++) { registerRenderer(geodeStone, i, BlockNames.GEODE_STONE); } } @SideOnly(Side.CLIENT) public static void registerRenderer(Block block, int meta, String file){ Item item = Item.getItemFromBlock(block); Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register( item, meta, new ModelResourceLocation(BeyondMod.MODID+":"+file, "inventory") ); } } Here's the block class for GeodeStone. public class BlockGeodeStone extends Block { public static final PropertyEnum<GeodeStoneVariant> variant = PropertyEnum.create("variant", GeodeStoneVariant.class); public BlockGeodeStone(String registryName){ super(Material.ROCK); setDefaultState(this.blockState.getBaseState().withProperty(variant, GeodeStoneVariant.SMOOTH)); setRegistryName(registryName); setUnlocalizedName(registryName); } public BlockStateContainer createBlockState(){ return new BlockStateContainer(this, new IProperty[]{variant}); } public int getMetaFromState(IBlockState state){ return state.getValue(variant).ordinal(); } public IBlockState getStateFromMeta(int meta){ return getDefaultState().withProperty(variant, GeodeStoneVariant.values()[meta]); } public int damageDropped(IBlockState blockState){ return getMetaFromState(blockState); } public void getSubBlocks(Item item, CreativeTabs tab, NonNullList<ItemStack> list){ for (int i = 0; i < GeodeStoneVariant.values().length; i++){ list.add(new ItemStack(item, 1, i)); } } public ItemStack getPickBlock(IBlockState state, RayTraceResult target, World world, BlockPos pos, EntityPlayer player){ return new ItemStack(this, 1, getMetaFromState(state)); } } This is the ItemBlockMetadata class I'm using. public class ItemBlockMetadata extends ItemBlock { public ItemBlockMetadata(Block block){ super(block); this.setHasSubtypes(true); } public String getUnlocalizedName(ItemStack stack){ return super.getUnlocalizedName(stack) + "_" + GeodeStoneVariant.values()[stack.getItemDamage()].toString().toLowerCase(); } public int getMetadata(int meta){ return meta; } public boolean placeBlockAt(ItemStack stack, EntityPlayer player, World world, BlockPos pos, EnumFacing side, float hitX, float hitY, float hitZ, IBlockState newState) { return super.placeBlockAt(stack, player, world, pos, side, hitX, hitY, hitZ, newState); } } This is the block state file for geode stone: { "variants": { "variant=smooth": { "model": "beyond:geode_stone_smooth" }, "variant=cobble": { "model": "beyond:geode_stone_cobble" }, "variant=brick": { "model": "beyond:geode_stone_brick" } } } And finally, what seems to be causing the issue: an item model for one of these variants. { "parent":"beyond:block/geode_stone_brick", "display": { "thirdperson": { "rotation": [ 10, -45, 170 ], "translation": [ 0, 1.5, -2.75 ], "scale": [ 0.375, 0.375, 0.375 ] } } } Now, for whatever reason, this doesn't give any errors related to missing/malformed files AT ALL. Nothing. It just refuses to load the item model for some reason. Sorry for the long winded post, I've been at this for a long time and I've tried nearly everything to no avail. Thanks guys.
-
[1.9] Make enchantment table not consume item?
iWasHere replied to iWasHere's topic in Modder Support
Alright, I tried this out. It looks like it had some effect on the inventory, the only problem being that the enchantment table itself ceases to function. It acts like the item in the enchanting slot is already enchanted, no options to enchant or anything, even with vanilla lapis in the table. Here's the code in the inventory class for getting the stack (where lapisGem is my item): @Override public ItemStack getStackInSlot(int index) { ItemStack stack = iInventory.getStackInSlot(index); if (stack.getItem().equals(RegisterItems.lapisGem)){ stack = new ItemStack(Items.dye,64,4); } return stack; } -
[1.9] Make enchantment table not consume item?
iWasHere replied to iWasHere's topic in Modder Support
Thanks! I'll try it once I get the chance. -
Hey all, I'm trying to make an item that basically acts like an infinite source of lapis lazuli. You can use it to dye wool/sheep without consuming the item. However, there seems to be one problem. Because the vanilla enchantment table uses lapis to enchant items since 1.8, you can use this item that cannot be consumed to enchant items. The problem is, the item is consumed when you enchant, as lapis normally is. I've tried to see if there's some sort of event for enchanting items in the table, and there isn't. I can't find any possible method to make it so the enchant table doesn't consume my item. I've done several searches, and I guess I'm the only one that's tried this (given, it's a pretty specific problem). Do you guys have any ideas on what I could do to solve this? Thanks for your time.
-
Oh crud, thanks! Good thing I checked back on here.
-
I know about lang files and all that, but I didn't know about I18n.format(), which seemed to solve my problem. Thanks!
-
When I try that, it just spits out event.slimerain.end, but in red/italics/whatever.
-
Hi, I can't seem to send a translated chat component to a client that has any formatting such as color or italics. I can send the translated chat message, but I can't apply a color like Aqua to it. I've tried doing things like putting the color format code directly into the lang file itself but that doesn't work. I can't find anything through google that would help me, but all that I could find that was relevant was this (http://www.minecraftforge.net/forum/index.php?topic=25566.0), but that info is either outdated or just plain doesn't work. Relevant code: Thanks in advance!
-
Using the latest version of Forge for 1.7.10 [10.13.3.1408]. I'm trying to make a mod that uses the redstoneflux api, but I want to be able to use Thermal Dynamics + Expansion with it for ease of testing, but putting the mods into eclipse/mods will crash the game. I tried this with many other mods like CodeChickenCore+NEI (the loader crashes at startup) and even Botania (which won't crash, but when I add Baubles it crashes). I'm not sure what to do, and considering I was able to get this to work in an earlier version of Forge [10.13.2.1291], I have no clue what I'm doing wrong. I tried to downgrade forge using the build.gradle file, but it didn't work, so I'm basically stuck until I can fix this. Thanks in advance. EDIT: totally reinstalled forge, tried adding the mods to be used in-game, same error.
-
Ah, thank you!
-
Ok, so this works: ItemStack head = new ItemStack(Items.skull,1,3); head.setTagCompound(new NBTTagCompound()); head.stackTagCompound.setString("SkullOwner", "iWasHere"); BUT if I were to try and set the vanilla "name" tag, the name of the item stays the same in-game. It's because the Name and Lore tags are under the "display" tag, which is a compound tag. Because of that, I can't access the vanilla Name and Lore tags. head.stackTagCompound.getCompoundTag("display").setString("Lore","It's special. Oh so special."); The above doesn't work. I have no clue how to proceed. EDIT: found out that there is a method for setting the display name of an itemstack. Still not sure about the lore though.
-
Nope, just on a standard ItemStack with a vanilla item (like a stick or something).
-
I've looked absolutely everywhere and I can't find a single answer to my question: how do I add values to compound nbt tags? I can do regular nbt tags like SkullOwner and the such, but not anything under "display", like "Name" or "Lore". How can I access/write to these tags? Thanks in advance.
-
Oooooohh boy. That kinda sucks for me, just started modding a couple days ago. I guess I'll just have to make it so entities hit by lightning drop my item. Almost never happens, but hey, that's what I gotta do I guess. Thanks guys.
-
How can I tell when/where a lightning bolt strikes? I've tried basically all of the events relating to entity spawning, and none work. The only even relating to lightning seems to be when entities are struck by it. Does anybody have any idea of what I could do? Thanks in advance.
-
Thanks! Works perfectly!
-
I made an enchantment that goes on chest plates. It lets you fly but does heavy durability damage while you're flying. However, once the durability on the plate reaches zero, the plate can still be worn and used, and the player must either [A) throw it out of their inventory B) get hit] to have it finally break. It's really kind of annoying. This is the code with the problem. Everything works just fine, but not getting the item to break/delete. if (ply.capabilities.isFlying) { if (chestplate.getMaxDamage() == chestplate.getItemDamage()) { --chestplate.stackSize; } else { chestplate.damageItem(1, ply); } } Does anyone have any ideas? Been stuck on this all day. Thanks in advanced.