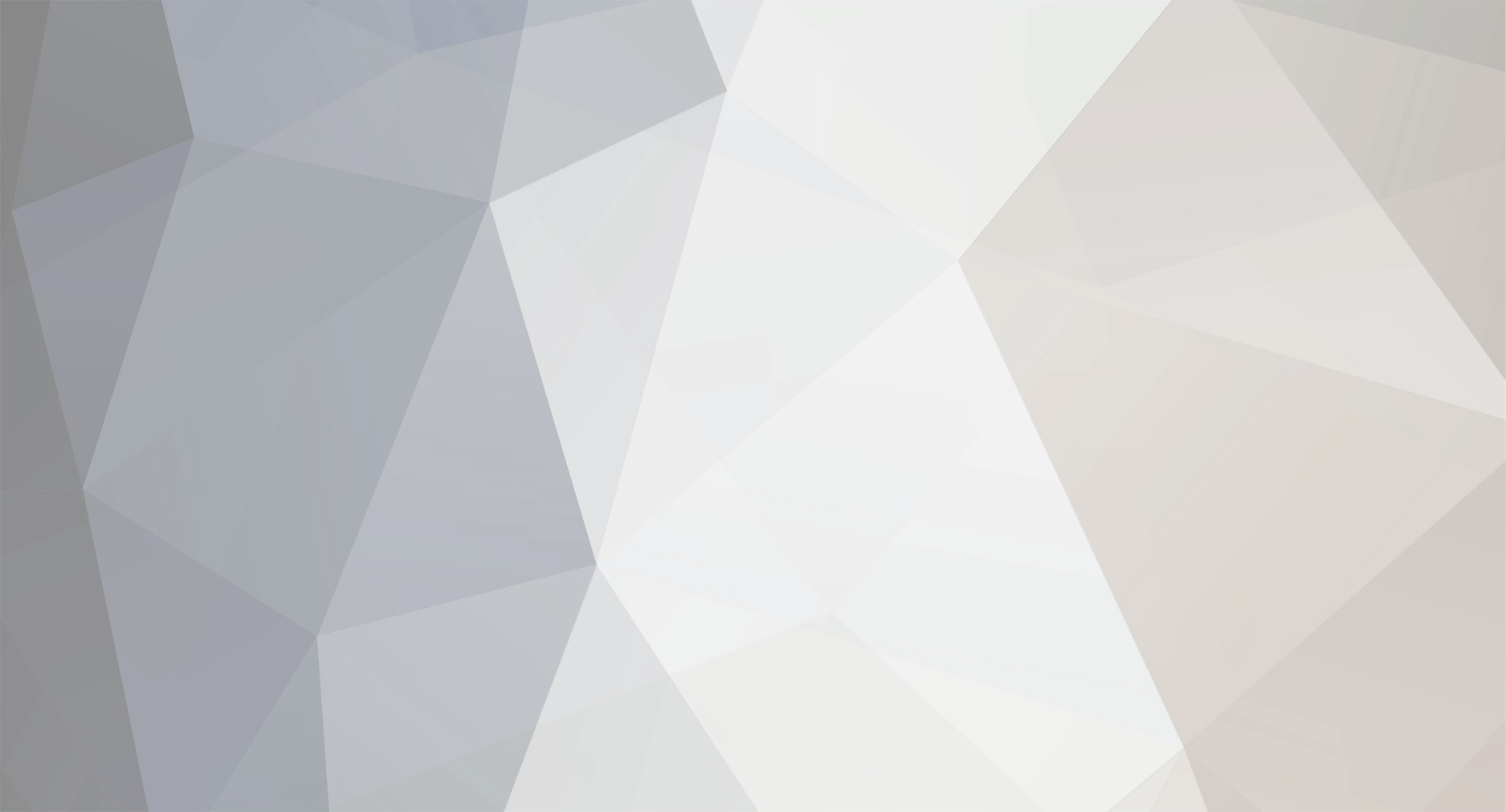
mysticalherobine
Members-
Posts
16 -
Joined
-
Last visited
Everything posted by mysticalherobine
-
BUMP, So I've gotten it working and all, but I fear for compatibility, what if another mod replaces the instance of the TileEntityItemStackRenderer? I've tried using an ItemStackTESR, but it deprecated and doesn't allow you to pass and item to check it's NBTCompounds. So I'm at a loss if I should stick to overwriting the default TileEntityItemStackRenderer, or somehow dealing with baked quads and all the "great" stuff.
-
Okay, so ignore everything beneath the end of the edit, it's now useless. What I did was created my own version of the TileEntityItemStackRenderer in Minecraft, EXCEPT that it adds an if statement for my shield. It provides the exact same functionality as the vanilla one, overwritting it's instance using: public static void init() { for (EnumDyeColor enumdyecolor : EnumDyeColor.values()) { SHULKER_BOXES[enumdyecolor.getMetadata()] = new TileEntityShulkerBox(enumdyecolor); } TileEntityItemStackRenderer.instance = new TileEntityItemStackRendererImpl(); } In my own class! Now I'm pretty sure this will break if other mods attempt the same thing, so maybe I should make an API allowing other mods to hook into this, since it makes shield rendering SUPER, and I mean SUPER easy. Should this be a thing, or is it to much? === END OF EDIT ============ Hey there! I'm trying to figure out how to layer a banner texture, similar to how Minecraft does it already. I've come to the conclusion that creating an IBakedModel in replace of the default one is best, which turned into this public class ModelShieldEmerald extends ModelBaker implements IBakedModel { private ModelShieldEmerald.ItemOverride itemOverride; public ModelShieldEmerald() { itemOverride = new ModelShieldEmerald.ItemOverride(Collections.EMPTY_LIST); } public List<BakedQuad> getQuads(IBlockState state, EnumFacing side, long rand) { List<BakedQuad> list = baseModel.getQuads(state, side, rand); System.out.println("Start"); for (BakedQuad quad : list) { int[] data = quad.getVertexData(); System.out.println(quad.getFormat().toString()); } return baseModel.getQuads(state, side, rand); } public boolean isAmbientOcclusion() { return baseModel.isAmbientOcclusion(); } public boolean isGui3d() { return baseModel.isGui3d(); } public boolean isBuiltInRenderer() { return baseModel.isBuiltInRenderer(); } public TextureAtlasSprite getParticleTexture() { return baseModel.getParticleTexture(); } public ItemOverrideList getOverrides() { return itemOverride; } public Pair<? extends IBakedModel, Matrix4f> handlePerspective( ItemCameraTransforms.TransformType cameraTransformType) { return Pair.of(this, baseModel.handlePerspective(cameraTransformType).getRight()); } public static class ItemOverride extends ItemOverrideList { public ItemOverride(List<net.minecraft.client.renderer.block.model.ItemOverride> overridesIn) { super(overridesIn); } public IBakedModel handleItemState(IBakedModel originalModel, ItemStack stack, @Nullable World world, @Nullable EntityLivingBase entity) { if (!stack.hasTagCompound()) return originalModel; return (IBakedModel) new ModelShieldEmerald().setBaseModel(originalModel); } } } I'm unsure of how to accomplish this, I really don't understand BakedQuads yet. But the other way, which seems impossible of doing is to go along the lines of what Minecraft does (except it doesn't work how I would expect it) The vanilla code goes from net.minecraft.client.renderer.RenderItem#renderItem(ItemStack, IBakedModel) public void renderItem(ItemStack stack, IBakedModel model) { if (!stack.isEmpty()) { GlStateManager.pushMatrix(); GlStateManager.translate(-0.5F, -0.5F, -0.5F); if (model.isBuiltInRenderer()) { GlStateManager.color(1.0F, 1.0F, 1.0F, 1.0F); GlStateManager.enableRescaleNormal(); TileEntityItemStackRenderer.instance.renderByItem(stack); } else { this.renderModel(model, stack); if (stack.hasEffect()) { this.renderEffect(model); } } GlStateManager.popMatrix(); } } to the TileEntityItemStackRenderer#renderByItem(ItemStack) - I think that is what it is doing... From there the things are all hard coded, but the shield is rendered as such within that class if (item == Items.SHIELD) { if (p_192838_1_.getSubCompound("BlockEntityTag") != null) { this.banner.setItemValues(p_192838_1_, true); Minecraft.getMinecraft().getTextureManager().bindTexture(BannerTextures.SHIELD_DESIGNS.getResourceLocation(this.banner.getPatternResourceLocation(), this.banner.getPatternList(), this.banner.getColorList())); } else { Minecraft.getMinecraft().getTextureManager().bindTexture(BannerTextures.SHIELD_BASE_TEXTURE); } GlStateManager.pushMatrix(); GlStateManager.scale(1.0F, -1.0F, -1.0F); this.modelShield.render(); GlStateManager.popMatrix(); } I'm really not sure what to do from here... I could attempt to layer the banner texture over top using UVs somehow (another topic I'm not sure how to accomplish) or get the item to correctly render similar to the Vanilla code. I have attempted the second option, but with odd results, meaning the shield doesn't render where it should have, nor is it rendering as the actual item. An help is appreciated, I can provide more details if necessary
-
Hey there, so I've recently succeeded in combining two BakedQuad sets using IBakedModel, but my problem now lies to adjusting them. So the way the item works it using it's base texture, then shrinking the texture of the selected item (Currently a placeholder Apple texture) into a smaller 4x4 size compared to the normal 16x16 size. I've come to find that the format for the quad texture location is 3 floats, each stored into 4 sections of the vertex data (I think). What I attempt to do is shrink the X and Y factors by 0.25, if those are even the two correct positions. What I'm doing just as a test to see what happens is as follows public List<BakedQuad> getQuads(IBlockState state, EnumFacing side, long rand) { if (side != null) return parent.getQuads(state, side, rand); List<BakedQuad> combined = new ArrayList(parent.getQuads(state, side, rand)); // Take additional item and compress quads List<BakedQuad> additonalShrinkQuads = new ArrayList(); System.out.println("New Set"); for (BakedQuad quad : additional.getQuads(state, side, rand)) { int[] vertexData = quad.getVertexData(); System.out.println(vertexData); vertexData[0] = (int) (vertexData[0] * 0.5); vertexData[4] = (int) (vertexData[4] * 0.5); vertexData[8] = (int) (vertexData[8] * 0.5); additonalShrinkQuads.add(new BakedQuad(vertexData, quad.getTintIndex(), quad.getFace(), quad.getSprite(), quad.shouldApplyDiffuseLighting(), quad.getFormat())); } combined.addAll(additonalShrinkQuads); return combined; } which works, other than the changing the additional quads (the item to display inside of the main item). This is a funny thing that happened from the above code an another snippet I tried to do Yeah, fun times, so any info into how to adjust the vertex data is welcome! Edit: I'd also like to know how to make sure the model is then held like a normal item as well, using the custom method seems to make it held in the weird awkward manner as seen in the pictures. Thanks again!
-
[1.12] [Solved] Get IBakedModel from ItemStack
mysticalherobine replied to mysticalherobine's topic in Modder Support
Bump, edited main post for a new question of sorts, but still related enough to not make a new one. -
EDIT: I solved how to make a custom renderer for the Item, but I need to render the selected item over it. I can't figure out how to do such a thing, any help is appreciated! Thanks in advance. OLD: Hey there! So my problem is that I don't quite understand how to dynamically render items using things such as IModel or IBakedModel. The task is pretty simple, I just want to render my item, then in a layer above, render the currently selected item from within the item's Inventory. Essentially, a backpack that allows you to scroll to a specific item, using shift-scroll, to place down blocks. But, for better or for worse, I can't quite get my head around how to use the classes to render the item in such a way. I assume that it wouldn't be to hard and I'm just over looking something. Thanks for any help in advance!
-
[1.11.2] [SOLVED] Changing variables Server-side only
mysticalherobine replied to Bektor's topic in Modder Support
First off, do you have a class that loads, saves, and stores all your config data? If not I would highly recommend you have one, it would be the place to store all your variables. Since you want to send data, try to create a PacketConfig class that handles sending data from Server->Client. In the packet, just define the variables inside of the packet that you want the server to be able to edit and send to the client side of things, e.g. MachineEnergyPerTick or MachineProgressSpeed. Then, just send the packet to the client, have them read the packet and change the values specified in the Config class to match. It wouldn't change the config file and would sync up the server to client. -
[1.12] Using Forge Energy (FE) - help still needed!
mysticalherobine replied to Sommanker's topic in Modder Support
I would highly recommend learning to use the RF Api by TeamCOFH. It is widely used and means you're mod would be much more compatible with others, which is always a plus. Though I've never worked with energy before, I would strongly suggest RF as the route you should take. There's more likely to be tutorials for the API compared to FE since it is so wide spread too. P.S. After some quick search I found the GitHub for the Redstone Flux API, which seems to include small implementations of how to use the basic system -
Nevermind, I was able to figure out that I can send NBTTagCompounds through packets, which I did to make sure all clients listening to the stereo had their TileEntity update with the correct inventory. Thank you diesieben07 for all your help and tips!
-
Sorry, that's not what I trying to get at, I've already done that it works fine for repeating that way. BUT I don't want it that way. Think of it this way: What if one client's resource pack changes "Block" a 5 minute song to a mere 1 minute "Nyan Cat Remix"? They have to wait 4 minutes until the song changes server side. How about changing modded songs as well with a resource pack? Or, what about the opposite, changing "Mellohi" to a one hour loop of "Epic Sax Guy"? That means the song would cut off way before the end came around. I know it's not a huge deal, but I want to make the mod work well for any end user, that's the goal I want to have in mind. So I don't simply want to loop through a list of songs server side, I've already done that and got it working marvelously. Instead I want to aim to all users, those who like other songs or want to play a modded song. So instead of using the server side event, I want to use the client side to play songs and loop through the inventory, which is why I need the TileEntity to sync it's contents.
-
Oh sorry about that, what I meant was that the server will still send a packet saying whether to play or stop songs, but since you mentioned about saying which song to play FIRST, I might include that in the packet later. BUT I did mean the client side will determine everything afterwards (which song to play and when), that's why I want to update the TileEntity to make sure the client has an update-to-date inventory for it. I don't know if this will help you sorta get what I'm trying to say: Firstly, the block is activated via Right-Clicking Secondly, a packet (From the server) is sent out saying whether to play/stop songs. Thirdly, the packet is read by the necessary clients. Fourthly, the packet calls upon the CLIENT'S TileEntity public void activateTileEntity(boolean playSongs) { if (playSongs) { ItemRecord record = (ItemRecord) contents.get(discLocationSlot).getItem(); world.playEvent((EntityPlayer) null, 1010, pos, Item.getIdFromItem(record)); } else { world.playEvent(1010, pos, 0); world.playRecord(pos, (SoundEvent) null); } which is a custom method to play/stop songs. The whole problem with this approach is that the TileEntity contents array private NonNullList<ItemStack> contents = NonNullList.withSize(14, ItemStack.EMPTY); may never have been updated, as seen when I have a disc in the block, leave the world, rejoin, and right click to play a sound. I can tell it is a sync issue because opening the gui, then right clicking works perfect every time.
-
Thank you, I'll look into making sure only certain players tracking the TileEntity will receive the packet. But my reason for not sending the sounds is they NEED to have an update. The stereo will loop through songs, using the client side song files, and get the effective tick count per song. This means that I cannot use just Vanilla song ticks (which I have already done, but was not satisfied with [It was hard coded]), I want to use resource locations to get the current song via possible resource packs and hopefully get other mods to work with it to. If I can't find another way to get the TileEntity to force sync the contents, then I'll probably just revert back to the previous state, though I was hoping to attempt this at the very least. Thank you in advance! Quick Edit: The TileEntity stores 14 Records inside a NonNullList<ItemStack> within it. The I'll most likely be going with what you said, so clients know which song to play first, plus a seed to make sure clients attempt to sync up song playing with a random.
-
Hey there, so the question seems generic and idiotic, but I couldn't seem to find a way to do this. So, what I'm attempting to do is send a packet of data to all clients, saying "Hey, I want this tile entity to start/stop playing songs." This works fine and dandy, but if the client hasn't opened the gui to see the inventory in the container, they never get the ItemRecord, which in turn means no song plays. Some insight into how it runs Client right clicks block to start/stop stereo, server gets this data and sends a packet to all clients saying whether to play songs or to stop playing songs. The client then using the TileEntity in the location of the block, via the Packet, to get it's inventory and play a disc. What ends up happening is that if a client hasn't opened the TileEntity via Shift-right clicking it, then the client has no inventory stored in the TileEntity. I'm not really to sure how to go about doing this, here's a snippet of the Packet call public boolean onBlockActivated(World worldIn, BlockPos pos, IBlockState state, EntityPlayer player, EnumHand hand, EnumFacing side, float hitX, float hitY, float hitZ) { if (worldIn.isRemote) return true; if (player.isSneaking()) player.openGui(VanillaExpansion.instance, ProxyGui.BLOCK_STEREO, worldIn, pos.getX(), pos.getY(), pos.getZ()); else PacketHandler.INSTANCE.sendToAll(new PacketStereo(pos, (isPlaying = !isPlaying))); return true; } If more info is needed I will provide it, but I just want to know the most efficient and practical to update clients when the block has been clicked, to make sure inventories are synced. I should be able to implement when the inventory is changed myself. All help is appreciated!
-
How are you activating the gui? Make sure the server side is calling it, not the client. When activating the block, does it include if (world.isRemote) return true; If not, that may be your problem
-
[1.12] ItemRecord Sound to InputStream
mysticalherobine replied to mysticalherobine's topic in Modder Support
Can you elaborate for me please? I though I was already using record.getSound() I was using the SoundEvent give by the above method to then get the resource location using record.getSound().getSoundName() Is that wrong for retrieving sound InputStreams? Am I supposed to use the sound event to get the location instead of using the getSoundName() method? -
Hey there everyone. I'm currently attempting to fetch the input stream for the sound file of a record. The problem is that every time I attempt to it throws an exception such as [02:24:49] [Server thread/INFO] [STDERR]: [de.mystic.vanillaexpansion.tileentity.TileEntityStereo:playCurrent:79]: java.io.FileNotFoundException: minecraft:record.mellohi [02:24:49] [Server thread/INFO] [STDERR]: [de.mystic.vanillaexpansion.tileentity.TileEntityStereo:playCurrent:79]: at net.minecraft.client.resources.FallbackResourceManager.getResource(FallbackResourceManager.java:69) [02:24:49] [Server thread/INFO] [STDERR]: [de.mystic.vanillaexpansion.tileentity.TileEntityStereo:playCurrent:79]: at net.minecraft.client.resources.SimpleReloadableResourceManager.getResource(SimpleReloadableResourceManager.java:65) [02:24:49] [Server thread/INFO] [STDERR]: [de.mystic.vanillaexpansion.tileentity.TileEntityStereo:playCurrent:79]: at de.mystic.vanillaexpansion.tileentity.TileEntityStereo.playCurrent(TileEntityStereo.java:75) [02:24:49] [Server thread/INFO] [STDERR]: [de.mystic.vanillaexpansion.tileentity.TileEntityStereo:playCurrent:79]: at de.mystic.vanillaexpansion.tileentity.TileEntityStereo.activateTileEntity(TileEntityStereo.java:59) [02:24:49] [Server thread/INFO] [STDERR]: [de.mystic.vanillaexpansion.tileentity.TileEntityStereo:playCurrent:79]: at de.mystic.vanillaexpansion.block.BlockStereo.onBlockActivated(BlockStereo.java:53) [02:24:49] [Server thread/INFO] [STDERR]: [de.mystic.vanillaexpansion.tileentity.TileEntityStereo:playCurrent:79]: at net.minecraft.server.management.PlayerInteractionManager.processRightClickBlock(PlayerInteractionManager.java:472) [02:24:49] [Server thread/INFO] [STDERR]: [de.mystic.vanillaexpansion.tileentity.TileEntityStereo:playCurrent:79]: at net.minecraft.network.NetHandlerPlayServer.processTryUseItemOnBlock(NetHandlerPlayServer.java:757) [02:24:49] [Server thread/INFO] [STDERR]: [de.mystic.vanillaexpansion.tileentity.TileEntityStereo:playCurrent:79]: at net.minecraft.network.play.client.CPacketPlayerTryUseItemOnBlock.processPacket(CPacketPlayerTryUseItemOnBlock.java:68) [02:24:49] [Server thread/INFO] [STDERR]: [de.mystic.vanillaexpansion.tileentity.TileEntityStereo:playCurrent:79]: at net.minecraft.network.play.client.CPacketPlayerTryUseItemOnBlock.processPacket(CPacketPlayerTryUseItemOnBlock.java:13) [02:24:49] [Server thread/INFO] [STDERR]: [de.mystic.vanillaexpansion.tileentity.TileEntityStereo:playCurrent:79]: at net.minecraft.network.PacketThreadUtil$1.run(PacketThreadUtil.java:21) [02:24:49] [Server thread/INFO] [STDERR]: [de.mystic.vanillaexpansion.tileentity.TileEntityStereo:playCurrent:79]: at java.util.concurrent.Executors$RunnableAdapter.call(Unknown Source) [02:24:49] [Server thread/INFO] [STDERR]: [de.mystic.vanillaexpansion.tileentity.TileEntityStereo:playCurrent:79]: at java.util.concurrent.FutureTask.run(Unknown Source) [02:24:49] [Server thread/INFO] [STDERR]: [de.mystic.vanillaexpansion.tileentity.TileEntityStereo:playCurrent:79]: at net.minecraft.util.Util.runTask(Util.java:53) [02:24:49] [Server thread/INFO] [STDERR]: [de.mystic.vanillaexpansion.tileentity.TileEntityStereo:playCurrent:79]: at net.minecraft.server.MinecraftServer.updateTimeLightAndEntities(MinecraftServer.java:795) [02:24:49] [Server thread/INFO] [STDERR]: [de.mystic.vanillaexpansion.tileentity.TileEntityStereo:playCurrent:79]: at net.minecraft.server.MinecraftServer.tick(MinecraftServer.java:740) [02:24:49] [Server thread/INFO] [STDERR]: [de.mystic.vanillaexpansion.tileentity.TileEntityStereo:playCurrent:79]: at net.minecraft.server.integrated.IntegratedServer.tick(IntegratedServer.java:192) [02:24:49] [Server thread/INFO] [STDERR]: [de.mystic.vanillaexpansion.tileentity.TileEntityStereo:playCurrent:79]: at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:589) [02:24:49] [Server thread/INFO] [STDERR]: [de.mystic.vanillaexpansion.tileentity.TileEntityStereo:playCurrent:79]: at java.lang.Thread.run(Unknown Source) The way I attempt to get the file is through: ItemRecord record = (ItemRecord) contents.get(0).getItem(); System.out.println(record.getSound().getRegistryName() + " : " + record.getSound().getSoundName()); try { InputStream stream = Minecraft.getMinecraft().getResourceManager().getResource(record.getSound().getSoundName()).getInputStream(); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } Which fails every time for some odd reason. Maybe I'm just overthinking or looking something, but the InputStream is never gotten from a failed resource name. Any help is very much appreciated. The reason I want to do this is for cross mod compatibility with other records added in to the game. (I want to use the file to get the length of the record)